socket通信 c#
时间: 2025-01-08 18:54:51 浏览: 1
### C# 中实现 Socket 通信
在 C# 中,`System.Net.Sockets.Socket` 类提供了用于创建套接字并执行网络操作的方法。下面展示了一个基本的客户端-服务器模型来说明如何使用 `Socket` 进行通信[^1]。
#### 创建服务器端程序
服务器负责监听来自客户端的消息请求,并作出响应:
```csharp
using System;
using System.Text;
using System.Net;
using System.Net.Sockets;
class Server {
public static void Main() {
int port = 9050; // 设置端口号
IPAddress localAddr = IPAddress.Parse("127.0.0.1"); // IP地址
// 建立一个 Tcp类型的终结点
var endpoint = new IPEndPoint(localAddr, port);
using (var listener = new Socket(endpoint.AddressFamily, SocketType.Stream, ProtocolType.Tcp)) {
try {
listener.Bind(endpoint); // 绑定到本地IP和端口上
listener.Listen(10); // 开始监听连接
Console.WriteLine("等待客户机连接...");
while(true){
var handler = listener.Accept(); // 接受新的连接
string data = null;
byte[] bytes = new byte[1024];
int bytesRec = handler.Receive(bytes);
data += Encoding.ASCII.GetString(bytes, 0, bytesRec);
Console.WriteLine($"收到消息:{data}");
string response = "你好";
byte[] msg = Encoding.ASCII.GetBytes(response);
handler.Send(msg); // 发送数据给已连接的客户端
handler.Shutdown(SocketShutdown.Both);
handler.Close();
}
} catch (Exception e) {
Console.WriteLine(e.ToString());
}
Console.WriteLine("\n按任意键退出...");
Console.ReadKey();
}
}
}
```
#### 客户端发送消息至服务端
此部分展示了怎样建立与上述服务器之间的TCP连接并向其传递字符串信息:
```csharp
using System;
using System.Text;
using System.Net.Sockets;
class Client {
public static void Main(){
string data = "测试消息";
byte[] msg = Encoding.ASCII.GetBytes(data);
// 要连接的服务端IP和端口
var ipPoint = new System.Net.IPEndPoint(IPAddress.Loopback, 9050);
using(var client = new Socket(AddressFamily.InterNetwork,
SocketType.Stream,
ProtocolType.Tcp)){
try{
client.Connect(ipPoint); // 尝试连接远程主机
Console.WriteLine("成功连接");
client.Send(msg); // 向目标机器上的应用程序发送数据报文
byte[] buffer = new byte[1024];
int size = client.Receive(buffer);
Console.WriteLine($"接收到来自服务器的信息:{Encoding.ASCII.GetString(buffer, 0, size)}");
client.Shutdown(SocketShutdown.Both);
client.Close();
}catch(Exception ex){
Console.WriteLine(ex.Message);
}
}
}
}
```
通过以上两个例子可以理解,在C#里利用内置库能够轻松构建起基于Socket协议的应用层通讯机制。
阅读全文
相关推荐




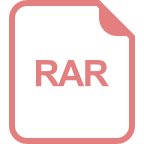


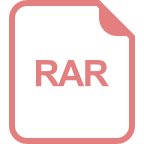








