Consider defining a bean of type 'java.util.Map' in your configuration.
时间: 2023-11-22 18:49:42 浏览: 29
这个错误通常是由于Spring框架无法找到所需的bean而引起的。如果你在代码中使用了一个类型为`java.util.Map`的bean,但是Spring容器中没有定义这个bean,就会出现这个错误。解决这个问题的方法是在Spring配置文件中定义一个类型为`java.util.Map`的bean。以下是一个示例配置文件:
```xml
<beans>
<bean id="myMap" class="java.util.HashMap">
<constructor-arg>
<map>
<entry key="key1" value="value1"/>
<entry key="key2" value="value2"/>
</map>
</constructor-arg>
</bean>
</beans>
```
这个配置文件定义了一个名为`myMap`的bean,它的类型是`java.util.HashMap`,并且包含两个键值对。你可以根据自己的需要修改这个配置文件,以满足你的具体需求。
相关问题
Consider defining a bean of type 'java.util.List' in your configuration.
To define a bean of type 'java.util.List' in your configuration, you can use the `@Bean` annotation along with the `@Configuration` annotation. Here is an example:
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.ArrayList;
import java.util.List;
@Configuration
public class MyConfiguration {
@Bean
public List<String> myListBean() {
List<String> myList = new ArrayList<>();
// add elements to the list if needed
return myList;
}
}
```
In this example, we define a bean named `myListBean` of type `List<String>`. You can modify the implementation to add elements or customize it according to your requirements.
Consider defining a bean of type 'java.util.concurrent.ThreadPoolExecutor' in your configuration.
To define a bean of type 'java.util.concurrent.ThreadPoolExecutor' in your configuration, you can use the @Bean annotation in a configuration class. Here's an example:
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.Executors;
@Configuration
public class ThreadPoolConfig {
@Bean
public ThreadPoolExecutor threadPoolExecutor() {
int corePoolSize = 10;
int maxPoolSize = 20;
int queueCapacity = 100;
return new ThreadPoolExecutor(corePoolSize, maxPoolSize, 0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<>(queueCapacity));
}
}
```
In this example, we define a bean named 'threadPoolExecutor' of type 'java.util.concurrent.ThreadPoolExecutor'. The ThreadPoolExecutor is initialized with a core pool size of 10, a maximum pool size of 20, and a queue capacity of 100. You can adjust these values according to your requirements.
相关推荐
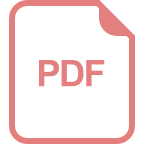
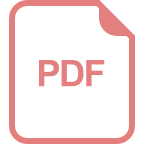












