数据结构C++稀疏矩阵转置
时间: 2023-11-14 13:58:14 浏览: 112
稀疏矩阵转置是将稀疏矩阵的行列互换得到新的矩阵。
在C语言中,可以使用三元组表示法来存储稀疏矩阵。三元组表示法包括三个数组:行索引数组、列索引数组和值数组。其中,行索引数组存储非零元素所在的行号,列索引数组存储非零元素所在的列号,值数组存储非零元素的值。
稀疏矩阵转置的基本思路是遍历原始稀疏矩阵,将每个非零元素的行列互换后存储到新的稀疏矩阵中。
下面是一个示例代码实现:
```c
#include<stdio.h>
#define MAX_TERMS 100
typedef struct {
int row;
int col;
int value;
} Element;
void transpose(Element a[], Element b[]) {
int n, m, terms, i, j, currentB;
n = a[0].row;
m = a[0].col;
terms = a[0].value;
b[0].row = m;
b[0].col = n;
b[0].value = terms;
if (terms > 0) {
currentB = 1;
for (j = 0; j < m; j++) {
for (i = 1; i <= terms; i++) {
if (a[i].col == j) {
b[currentB].row = a[i].col;
b[currentB].col = a[i].row;
b[currentB].value = a[i].value;
currentB++;
}
}
}
}
}
int main() {
int n, m, i, j, count = 1;
printf("Enter the number of rows and columns: ");
scanf("%d %d", &n, &m);
Element a[MAX_TERMS], b[MAX_TERMS];
a[0].row = n;
a[0].col = m;
printf("Enter the elements of the matrix: \n");
for (i = 0; i < n; i++) {
for (j = 0; j < m; j++) {
int element;
scanf("%d", &element);
if (element != 0) {
a[count].row = i;
a[count].col = j;
a[count].value = element;
count++;
}
}
}
transpose(a, b);
printf("\nOriginal sparse matrix:\n");
for (i = 0; i <= a[0].value; i++) {
printf("%d\t%d\t%d\n", a[i].row, a[i].col, a[i].value);
}
printf("\nTransposed sparse matrix:\n"); for (i = 0; i <= b[0].value; i++) {
printf("%d\t%d\t%d\n", b[i].row, b[i].col, b[i].value);
}
return 0;
}
```
这段代码中,我们首先定义了一个 `Element` 结构体来表示稀疏矩阵的非零元素。然后,使用 `transpose` 函数来实现稀疏矩阵的转置操作。最后,在 `main` 函数中,我们可以输入稀疏矩阵的行列数和元素,并输出原始稀疏矩阵和转置后的稀疏矩阵。
希望这段代码能帮助到你!如果有任何问题,请随时向我提问。
阅读全文
相关推荐
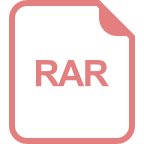
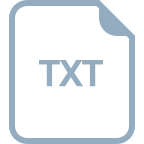
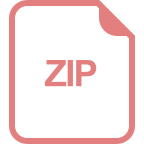
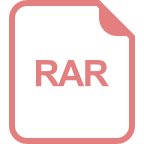
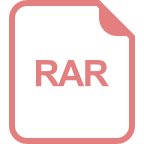
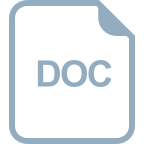
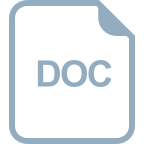
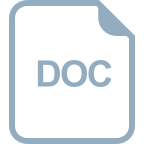
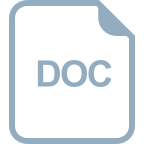
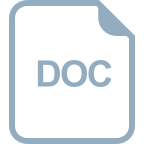
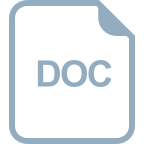

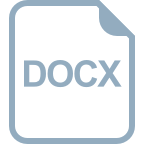
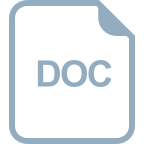
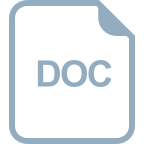
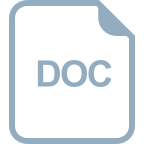