使用vue前端框架实现简单的用户信息管理程序。可自行设计API,采用axios方式模拟后端的接口和数据。
时间: 2024-04-10 08:33:32 浏览: 15
好的,我可以帮助你实现一个简单的用户信息管理程序。首先,我们需要设置一个后端接口来处理用户信息的增删改查操作。我们可以使用 JSONPlaceholder 这个在线模拟API来模拟后端接口和数据。
首先,在 Vue 项目中安装 axios,可以使用以下命令:
```
npm install axios
```
然后,我们可以创建一个 UserList.vue 组件来展示用户列表,并且使用 axios 来获取用户数据。在该组件中,我们可以使用 `created` 钩子函数来在组件创建时获取用户列表数据,并将其保存在 `users` 数组中。
```vue
<template>
<div>
<h1>用户列表</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>邮箱</th>
</tr>
</thead>
<tbody>
<tr v-for="user in users" :key="user.id">
<td>{{ user.id }}</td>
<td>{{ user.name }}</td>
<td>{{ user.email }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
users: []
};
},
created() {
this.fetchUsers();
},
methods: {
fetchUsers() {
axios.get('https://jsonplaceholder.typicode.com/users')
.then(response => {
this.users = response.data;
})
.catch(error => {
console.log(error);
});
}
}
};
</script>
```
接下来,我们可以在 App.vue 中使用 UserList 组件来展示用户列表。
```vue
<template>
<div id="app">
<UserList />
</div>
</template>
<script>
import UserList from './components/UserList.vue';
export default {
components: {
UserList
}
};
</script>
```
最后,我们需要在 main.js 中引入 Vue 和 App 组件,并创建一个 Vue 实例。
```javascript
import Vue from 'vue';
import App from './App.vue';
new Vue({
render: h => h(App),
}).$mount('#app');
```
现在,你可以通过运行你的 Vue 项目来查看用户列表了。在终端中执行以下命令:
```
npm run serve
```
这样就完成了一个简单的用户信息管理程序,使用了 Vue 前端框架和 axios 模拟后端接口和数据。
相关推荐
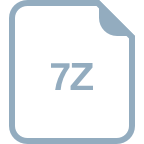
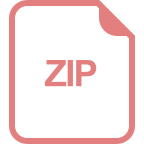
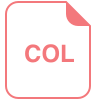













