对字符串的基数排序java
时间: 2024-12-15 18:13:21 浏览: 3
基数排序是一种非比较整数排序算法,它通过按照数字的位数,从低位到高位依次排序。在Java中,基数排序通常用于对整数数组进行排序,尤其适用于那些位数固定的情况,因为可以利用位操作简化过程。
以下是基本的基数排序 Java 实现步骤:
1. **确定最大数和位数**:首先找到待排序数组中的最大数,计算出需要排序的位数。
2. **设置桶(Bucket)数组**:创建一个长度为10(假设最多只处理0-9的十进制数)的桶数组,每个元素都是一个空列表。
3. **分桶阶段**:对于每一位,将当前位对应的值放入相应的桶中。例如,第一位是最低位,就看每位数字是0-9中的哪一位,放入对应的桶。
4. **收集阶段**:逐个取出每个桶中的元素,按顺序合并到结果数组中。
5. **重复以上步骤**:如果还有更高位未处理,就继续上述步骤,直到所有位都排序完毕。
```java
public class RadixSort {
public static void radixsort(int[] nums) {
int max = getMax(nums);
for (int exp = 1; max / exp > 0; exp *= 10) {
countingSort(nums, exp);
}
}
private static int getMax(int[] nums) {
int max = Integer.MIN_VALUE;
for (int num : nums) {
if (num > max) {
max = num;
}
}
return max;
}
// 计数排序辅助方法
private static void countingSort(int[] nums, int exp) {
int[] count = new int[10];
for (int num : nums) {
count[(num / exp) % 10]++;
}
for (int i = 1; i < count.length; i++) {
count[i] += count[i - 1];
}
List<Integer> output = new ArrayList<>();
for (int num : nums) {
output.add(count[(num / exp) % 10]--);
}
System.arraycopy(output.toArray(), 0, nums, 0, nums.length);
}
}
```
阅读全文
相关推荐
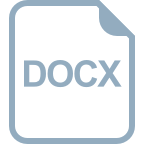
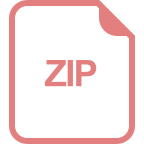
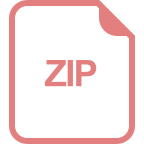

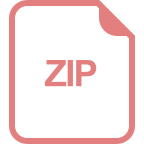
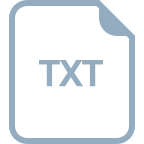
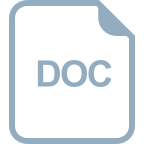
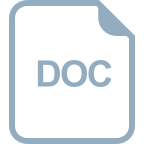
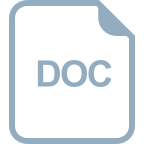
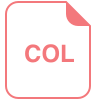

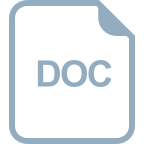
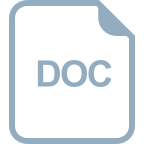
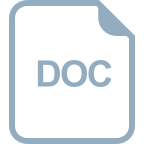
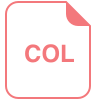


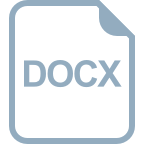