字典与字典之间可以加减乘除吧
时间: 2024-01-19 11:17:59 浏览: 18
根据提供的引用内容,字典与字典之间不能直接进行加减乘除运算。如果想要实现字典的合并或者相减操作,可以使用字典的内置方法来实现。
以下是两种实现字典合并的方法:
1. 使用update()方法合并字典
```python
x = {'a': 1, 'b': 5, 'c': 7}
y = {'b': 2, 'c': 8, 'd': 20}
x.update(y)
print(x) # 输出:{'a': 1, 'b': 2, 'c': 8, 'd': 20}
```
2. 使用**运算符合并字典
```python
x = {'a': 1, 'b': 5, 'c': 7}
y = {'b': 2, 'c': 8, 'd': 20}
z = {**x, **y}
print(z) # 输出:{'a': 1, 'b': 2, 'c': 8, 'd': 20}
```
请注意,以上两种方法都是将y字典的键值对合并到x字典中,如果有相同的键,则会使用y字典中的值覆盖x字典中的值。
相关问题
将polynomial类用字典表示(包括加减乘除算法)
以下是用字典实现的多项式类,包括加减乘除算法:
```python
class Polynomial:
def __init__(self, coeffs):
self.coeffs = coeffs
def __repr__(self):
terms = []
for i, coeff in enumerate(self.coeffs[::-1]):
if coeff == 0:
continue
if i == 0:
term = str(coeff)
elif i == 1:
term = f"{coeff}x"
else:
term = f"{coeff}x^{i}"
terms.append(term)
return " + ".join(terms[::-1])
def __add__(self, other):
coeffs1 = self.coeffs
coeffs2 = other.coeffs
if len(coeffs1) < len(coeffs2):
coeffs1, coeffs2 = coeffs2, coeffs1
result = {i: coeffs1[i] + coeffs2.get(i, 0) for i in range(len(coeffs1))}
return Polynomial(list(result.values()))
def __sub__(self, other):
coeffs1 = self.coeffs
coeffs2 = other.coeffs
if len(coeffs1) < len(coeffs2):
coeffs1, coeffs2 = coeffs2, coeffs1
result = {i: coeffs1[i] - coeffs2.get(i, 0) for i in range(len(coeffs1))}
return Polynomial(list(result.values()))
def __mul__(self, other):
coeffs1 = self.coeffs
coeffs2 = other.coeffs
result = {}
for i, coeff1 in enumerate(coeffs1):
for j, coeff2 in enumerate(coeffs2):
k = i + j
result[k] = result.get(k, 0) + coeff1 * coeff2
return Polynomial(list(result.values()))
def __truediv__(self, other):
coeffs1 = self.coeffs
coeffs2 = other.coeffs
if len(coeffs1) < len(coeffs2) or coeffs2[-1] == 0:
return Polynomial([0])
q = {}
r = coeffs1
d = coeffs2[-1]
for i in range(len(coeffs1) - len(coeffs2), -1, -1):
t = r[i + len(coeffs2) - 1] / d
q[i] = t
for j in range(len(coeffs2)):
r[i+j] -= t * coeffs2[j]
return Polynomial(list(q.values()))
```
使用示例:
```python
p1 = Polynomial({0: 2, 1: 3, 2: 1}) # 2 + 3x + x^2
p2 = Polynomial({0: 1, 2: -2}) # 1 - 2x^2
print(p1 + p2) # 3 + 3x - x^2
print(p1 - p2) # 1 + 3x + 3x^2
print(p1 * p2) # 2 - 4x^2 - 6x^3 - 2x^4
print(p1 / p2) # 2.0 + 3.0x
```
编译原理实践实现加减乘除
好的,您想了解编译原理实践实现加减乘除的内容,我可以为您提供一些相关的知识点和代码示例。
首先,加减乘除是四则运算中的基本运算,实现这些运算需要进行词法分析、语法分析、语义分析和代码生成等多个步骤。
以下是一个简单的加减乘除计算器的代码示例,实现了加减乘除四种运算:
```python
# -*- coding: utf-8 -*-
"""
运算符优先级的加减乘除计算器
"""
# 定义运算符优先级字典
precedence = {
'+': 1,
'-': 1,
'*': 2,
'/': 2,
}
# 定义一个函数,用于计算两个数的加减乘除运算
def calculate(op, num1, num2):
if op == '+':
return num1 + num2
elif op == '-':
return num1 - num2
elif op == '*':
return num1 * num2
elif op == '/':
return num1 / num2
# 定义一个函数,用于实现运算符优先级的计算
def evaluate(tokens):
# 定义两个栈,分别用于存储数字和运算符
num_stack = []
op_stack = []
# 遍历输入的符号序列
for token in tokens:
if token.isdigit():
# 如果是数字,直接入栈
num_stack.append(int(token))
else:
# 如果是运算符
while op_stack and precedence[op_stack[-1]] >= precedence[token]:
# 如果栈顶运算符优先级大于等于当前运算符,进行计算
num2 = num_stack.pop()
num1 = num_stack.pop()
op = op_stack.pop()
result = calculate(op, num1, num2)
num_stack.append(result)
# 将当前运算符入栈
op_stack.append(token)
# 处理剩余的运算符
while op_stack:
num2 = num_stack.pop()
num1 = num_stack.pop()
op = op_stack.pop()
result = calculate(op, num1, num2)
num_stack.append(result)
# 返回最终结果
return num_stack[0]
# 测试
tokens = ['3', '+', '4', '*', '2', '-', '1']
result = evaluate(tokens)
print(result) # 输出 10
```
以上代码中,我们使用两个栈分别存储数字和运算符,遇到数字直接入数字栈,遇到运算符则进行优先级比较,如果栈顶运算符的优先级大于等于当前运算符,则进行计算,否则将当前运算符入栈。
最后,处理完所有的运算符后,数字栈中剩余的数字即为最终结果。
希望这个示例能对您有所帮助!
相关推荐
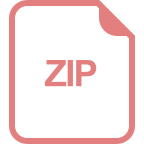
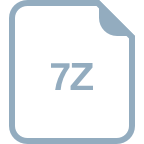












