帮我用C语言生成建立一个长度为n单链表的代码,通过赋值方法确定数据
时间: 2024-10-14 09:12:48 浏览: 35
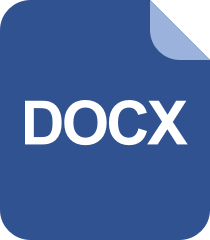
用C语言创建一个单链表的方法
在C语言中,你可以创建一个结构体表示链表节点,然后编写函数来创建、初始化并赋值一个新的链表。以下是一个简单的例子,假设我们有一个`ListNode`结构体,包含一个整数`data`和指向下一个节点的指针`next`:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
// 创建新节点并分配内存
ListNode* createNode(int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = NULL; // 初始化为NULL,表示当前节点没有后续节点
return newNode;
}
// 功能:创建一个长度为n的单链表,并通过循环赋值
ListNode* createLinkedList(int n, int startValue) {
ListNode* head = createNode(startValue); // 创建头节点
for (int i = 1; i < n; i++) { // 循环创建剩余节点
ListNode* newNode = createNode(i + startValue);
if (head != NULL) {
newNode->next = head->next; // 将新节点连接到上一个节点的尾部
head->next = newNode;
} else {
printf("Error: Cannot create list with less than two nodes.\n");
return NULL;
}
}
return head;
}
// 打印链表
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
int n, startValue;
printf("Enter the length of the list and the starting value: ");
scanf("%d %d", &n, &startValue);
ListNode* myList = createLinkedList(n, startValue);
if (myList != NULL) {
printList(myList);
}
return 0;
}
```
在这个示例中,用户输入链表的长度`n`和起始值`startValue`,程序会创建一个长度为`n`的链表,从`startValue`开始递增。
阅读全文
相关推荐
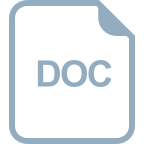

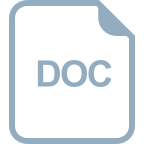
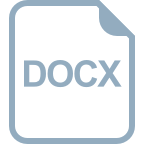
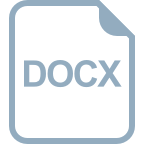
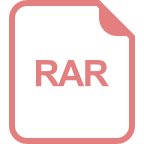
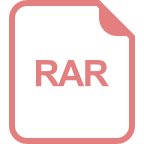
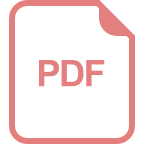
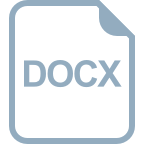
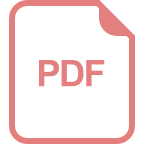
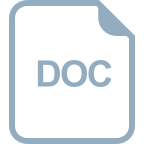
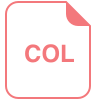
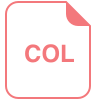
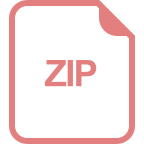
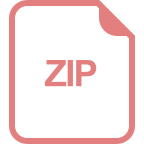
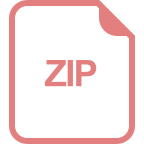