c语言输入一个日期,求该日期距离当年最后一天的天数。例如2016年12月10日,距离2016年底还有21天. 要求用switch语句实现。 输入 输入某年某月某日 输出 输出距离最后一天还有多少天
时间: 2024-09-30 12:07:59 浏览: 9
在C语言中,你可以使用`switch`语句配合一些基本的日期计算逻辑来实现这个功能。首先,需要获取用户的输入并转换成整型表示月份和日期。然后,考虑到二月有特殊规则(闰年有29天,非闰年有28天),我们还需要判断当前是否为闰年。以下是一个简单的示例:
```c
#include <stdio.h>
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int days_in_month(int month, int year) {
switch(month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31;
case 4: case 6: case 9: case 11:
return 30;
case 2:
if (is_leap_year(year)) {
return 29;
} else {
return 28;
}
default:
return -1; // 输入错误,不应到达这里
}
}
int main() {
int year, month, day, total_days;
printf("请输入年份、月份和日期(格式如:2016 12 10): ");
scanf("%d %d %d", &year, &month, &day);
total_days = day;
total_days += days_in_month(month, year) - 1; // 减去本月已经过去的天数
total_days += is_leap_year(year) ? 1 : 0; // 考虑到二月特殊情况
printf("从%d-%02d-%02d到年底还有 %d 天.\n", year, month, day, total_days);
return 0;
}
```
当用户运行此程序时,它会提示输入日期,然后输出距离该年度结束的剩余天数。
相关推荐
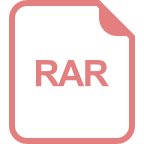
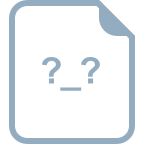
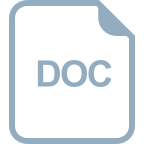














