如何使用C语言编程来计算每年的研究生入学考试(考研)的具体日期?
时间: 2024-10-24 17:03:14 浏览: 23
在C语言中,精确地计算每年的研究生入学考试(考研)日期通常需要考虑中国高考的日期以及研究生招生的时间安排。考研一般是在每年的12月份的第一个和第二个周末举行。由于这个时间相对固定,你可以创建一个函数,接收当前年份作为输入,然后根据已知的规律来推算出考研的具体日期。
以下是一个简单的示例,假设考研总是在12月的第一个周末:
```c
#include <stdio.h>
#include <time.h>
#define MONTH_LENGTH[12] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31} // 不考虑闰年
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
void calculate_mcat_date(int year) {
time_t now;
struct tm *date_info;
time(&now);
date_info = localtime(&now);
int current_month = date_info->tm_mon + 1; // 从0开始计数
if (current_month > 12) {
current_month -= 12;
++year;
}
if (is_leap_year(year) && current_month == 2 && date_info->tm_mday == 29) {
// 如果是闰年的2月29日,那么下个月的第一天就是考研的日子
date_info->tm_mday = 1;
} else {
date_info->tm_mday = 1; // 否则,直接取第一个周末
}
// 添加考研周日(一般是周末的第一天)
if (date_info->tm_wday != 6 && date_info->tm_wday != 0) {
++date_info->tm_mday; // 跳过非周末
}
printf("考研日期:%d-%02d-%02d\n", year, date_info->tm_mon + 1, date_info->tm_mday);
}
int main() {
int user_year;
printf("请输入你想查询的年份:");
scanf("%d", &user_year);
calculate_mcat_date(user_year);
return 0;
}
```
这个示例假设每年的1月1日都是星期一,实际的日期可能会因为历法调整而有所偏差,如果你需要更准确的结果,可能需要引入更复杂的历法学算法。
阅读全文
相关推荐
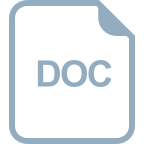
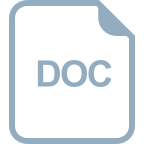
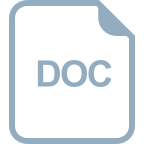
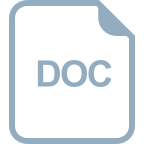
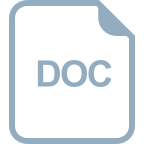
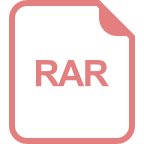
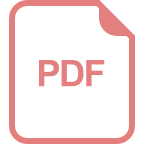
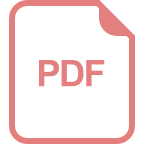
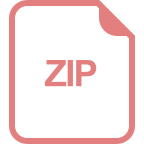
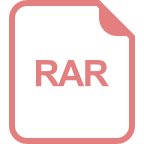
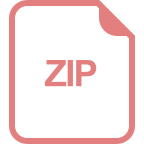
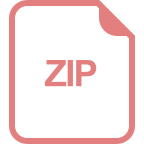
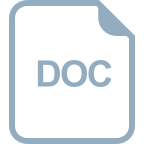
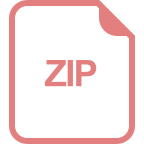
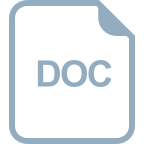
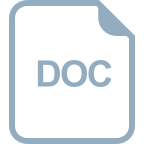
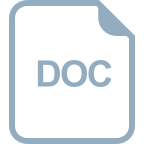
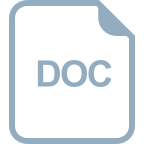
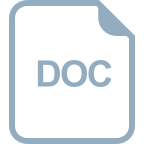