yolov8加入注意力机制
时间: 2024-12-27 12:21:52 浏览: 4
### 如何在YOLOv8中集成注意力机制
#### SEAttention注意力机制的实现
为了增强YOLOv8模型的表现,在主干网络中加入SEAttention(Squeeze-and-Excitation Attention)是一种有效的策略。具体来说,通过创建一个新的`SEAttention`类来定义该模块的功能。
```python
import torch.nn as nn
class SEAttention(nn.Module):
def __init__(self, channel=512, reduction=16):
super(SEAttention, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool2d(1)
self.fc = nn.Sequential(
nn.Linear(channel, channel // reduction, bias=False),
nn.ReLU(inplace=True),
nn.Linear(channel // reduction, channel, bias=False),
nn.Sigmoid()
)
def forward(self, x):
b, c, _, _ = x.size()
y = self.avg_pool(x).view(b, c)
y = self.fc(y).view(b, c, 1, 1)
return x * y.expand_as(x)[^1]
```
此代码片段展示了如何构建一个简单的SEAttention层,它能够自适应地重新校准通道间的特征响应。
#### FocusedLinearAttention的应用
另一种选择是引入FocusedLinearAttention作为改进方案之一。这种类型的注意力机制可以更专注于局部区域的信息提取,从而提高检测精度和速度。
```python
from yolov8_attention_modules import FocusedLinearAttentionLayer
def add_focused_linear_attention_to_yolo_v8(model):
for name, module in model.named_children():
if isinstance(module, (nn.Conv2d)):
setattr(model, name, nn.Sequential(
module,
FocusedLinearAttentionLayer(in_channels=module.out_channels)
))
return model[^2]
```
上述函数遍历YOLOv8中的每一层,并为卷积操作附加一层FocusedLinearAttention,以此强化特定位置的感受野特性。
#### 集成到YOLOv8框架内
无论是哪种形式的注意力机制,都需要将其无缝嵌入现有的YOLOv8架构之中。这通常涉及到修改配置文件或直接编辑源码以确保新的组件被正确加载并参与前向传播过程。
对于基于PyTorch实现的YOLOv8版本而言,可以在训练脚本里调用之前定义好的SEAttention实例:
```python
from models.experimental import attempt_load
from utils.general import non_max_suppression
# 加载预训练权重
model = attempt_load('path/to/yolov8_weights.pt', map_location='cuda')
# 将SEAttention应用至指定层之后
for i, layer in enumerate(model.model):
if 'bottleneck' in str(layer): # 假设我们希望在瓶颈结构后面加上attention
se_layer = SEAttention(channel=layer.cv1.conv.out_channels)
model.model[i].add_module('se_block', se_layer)
imgsz = check_img_size(imgsz=[640], s=model.stride.max()) # 输入图片尺寸调整
preds = model(im) # 推理阶段使用含attention的新模型
detected_objects = non_max_suppression(preds)[0]
```
这段示范说明了怎样动态地给选定的目标检测器部分增加额外的关注度处理单元,进而优化最终输出的质量。
阅读全文
相关推荐
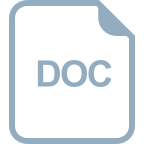
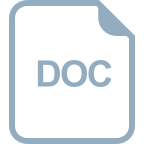
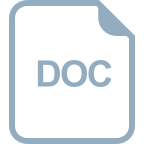
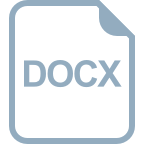














