void *turn_off_gps(void *args){
时间: 2023-12-15 12:01:43 浏览: 33
这段代码是一个函数的定义,函数名为turn_off_gps,参数类型为void指针args。在C语言中,void *表示一个指针,可以指向任何类型的数据。函数的功能是关闭GPS模块,参数args可能是用来传递一些关闭GPS模块的具体参数或者信息。
函数定义中的void *args表明这个函数没有返回值,因为void代表无类型,所以可以指向任何类型的数据。在函数体内部,可能会根据参数args的具体类型来进行关闭GPS模块的操作,例如关闭GPS模块的具体指令或者需要关闭的GPS模块的地址等。
这个函数的作用是在某些需要关闭GPS模块的地方调用,通过传入参数args来实现关闭GPS模块的操作。调用该函数的地方可能是在程序需要节省能量或者关闭GPS功能的时候,比如在设备休眠时或者不需要位置服务的时候。
总的来说,这段代码定义了一个关闭GPS模块的函数,通过传入void指针参数args来实现关闭GPS模块的具体操作。函数的具体功能和参数args传入的具体信息还需要根据实际情况来具体实现。
相关问题
java 管理员命令模式_java中命令模式详解和使用方法
Java中的命令模式是一种行为型设计模式,它将请求封装成对象,并将发出请求的对象和执行请求的对象分开。这种模式的核心思想是将命令的请求者与处理者解耦,从而实现松耦合的设计。
以下是Java中命令模式的详解和使用方法:
1. 定义命令接口
首先需要定义一个命令接口,该接口包含一个执行方法,该方法用于执行命令。
```
public interface Command {
void execute();
}
```
2. 定义具体命令类
接下来需要定义具体的命令类,每个命令类实现命令接口,并将命令的执行者作为参数传递给构造函数。
```
public class LightOnCommand implements Command {
private Light light;
public LightOnCommand(Light light) {
this.light = light;
}
public void execute() {
light.turnOn();
}
}
public class LightOffCommand implements Command {
private Light light;
public LightOffCommand(Light light) {
this.light = light;
}
public void execute() {
light.turnOff();
}
}
```
3. 定义命令调用者
接下来需要定义命令调用者,该调用者包含一个执行命令的方法,并将命令作为参数传递给该方法。
```
public class RemoteControl {
private Command command;
public void setCommand(Command command) {
this.command = command;
}
public void pressButton() {
command.execute();
}
}
```
4. 使用命令模式
最后,我们可以使用命令模式来控制电灯的开关。
```
public class Client {
public static void main(String[] args) {
Light light = new Light();
Command lightOnCommand = new LightOnCommand(light);
Command lightOffCommand = new LightOffCommand(light);
RemoteControl remoteControl = new RemoteControl();
remoteControl.setCommand(lightOnCommand);
remoteControl.pressButton();
remoteControl.setCommand(lightOffCommand);
remoteControl.pressButton();
}
}
```
以上就是Java中命令模式的详解和使用方法。在实际应用中,命令模式常常被用于撤销和重做功能的实现,以及异步处理任务等场景。
Java实现2、通过JAVA程序的面向接口编程的设计思想,模拟计算机使用USB接口驱动设备的过程。要求: ①定义USB接口,接口中有turnOn( )启动设备的方法,和turnOff( )关闭设备的方法; ②定义Mouse鼠标、KeyBoard键盘、Camera摄像头类实现USB接口; ③定义计算机Computer类 powerOn( )开机,开机前需要加载所有的USB设备;powerOff( )关机,关机前需要弹出所有的USB设备。 模拟开机输出:鼠标启动了 键盘启动了 摄像头启动了 计算机开机成功 模拟关机输出:鼠标关闭了 键盘关闭了 摄像头关闭了 计算机关机成功
好的,根据您的要求,我来为您编写这个程序。下面是实现代码:
首先,定义USB接口,包含启动和关闭设备的方法:
```java
interface USB {
void turnOn();
void turnOff();
}
```
然后,定义Mouse、Keyboard和Camera类,实现USB接口:
```java
class Mouse implements USB {
public void turnOn() {
System.out.println("鼠标启动了");
}
public void turnOff() {
System.out.println("鼠标关闭了");
}
}
class Keyboard implements USB {
public void turnOn() {
System.out.println("键盘启动了");
}
public void turnOff() {
System.out.println("键盘关闭了");
}
}
class Camera implements USB {
public void turnOn() {
System.out.println("摄像头启动了");
}
public void turnOff() {
System.out.println("摄像头关闭了");
}
}
```
接下来,定义计算机Computer类,包含开机和关机方法,以及USB设备列表:
```java
class Computer {
private List<USB> devices = new ArrayList<>();
public void powerOn() {
Mouse mouse = new Mouse();
Keyboard keyboard = new Keyboard();
Camera camera = new Camera();
devices.add(mouse);
devices.add(keyboard);
devices.add(camera);
for (USB device : devices) {
device.turnOn();
}
System.out.println("计算机开机成功");
}
public void powerOff() {
for (USB device : devices) {
device.turnOff();
}
System.out.println("计算机关机成功");
}
}
```
最后,我们可以在主函数中进行测试:
```java
public class Main {
public static void main(String[] args) {
Computer computer = new Computer();
computer.powerOn();
System.out.println("----------------------------");
computer.powerOff();
}
}
```
运行结果如下:
```
鼠标启动了
键盘启动了
摄像头启动了
计算机开机成功
----------------------------
鼠标关闭了
键盘关闭了
摄像头关闭了
计算机关机成功
```
希望这个程序能够满足您的要求。如果您有任何疑问或建议,请随时告诉我。
相关推荐
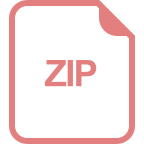
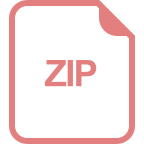
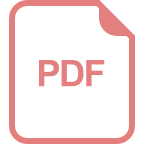












