3d粒子爱心动态动画代码
时间: 2024-09-22 13:09:35 浏览: 81
创建一个三维的爱心动态粒子动画需要一定的编程技巧,通常会涉及到WebGL、Three.js这样的JavaScript库,以及一些基本的几何形状和动画原理。这里是一个简单的示例,展示如何用Three.js制作一个基础的3D爱心粒子系统:
```javascript
// 引入Three.js库
import * as THREE from 'three';
// 创建一个场景
const scene = new THREE.Scene();
// 创建一个相机
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// 创建一个渲染器并设置它的大小
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// 创建一个粒子系统
const particles = [];
for (let i = 0; i < 1000; i++) {
const particle = new THREE.Points(
new THREE.Geometry(),
new THREE.PointsMaterial({ color: Math.random() * 0xffffff, size: 1 })
);
particle.position.x = Math.random() * 2 - 1;
particle.position.y = Math.random() * 2 - 1;
particle.position.z = Math.random() * 2 - 1;
particle.rotation.x = Math.random() * Math.PI;
particle.rotation.y = Math.random() * Math.PI;
particle.rotation.z = Math.random() * Math.PI;
scene.add(particle);
particles.push(particle);
}
// 定义爱心几何体
function heartGeometry(radius) {
// 这里只是一个简化的爱心几何,实际可以使用更复杂的模型
const vertices = [
new THREE.Vector3(-radius, radius * 0.5, 0),
new THREE.Vector3(radius, radius * 0.5, 0),
new THREE.Vector3(0, -radius, 0),
new THREE.Vector3(0, radius, 0)
];
const indices = [
0, 1, 2,
2, 3, 0
];
return new THREE.BufferGeometry().setFromPoints(vertices).setIndex(new THREE.BufferAttribute(indices, 1));
}
// 创建爱心点云
const heartCloud = new THREE.Points(heartGeometry(0.2), new THREE.PointsMaterial({ size: 0.02 }));
scene.add(heartCloud);
// 渲染和动画更新
function animate() {
requestAnimationFrame(animate);
for (let i = 0; i < particles.length; i++) {
particles[i].position.lerp(heartCloud.position, 0.05); // 模拟爱心吸引粒子
particles[i].rotation.x += 0.01;
particles[i].rotation.y += 0.01;
particles[i].rotation.z += 0.01;
}
renderer.render(scene, camera);
}
animate();
```
这个例子创建了一个随机分布的粒子群,并将它们慢慢拉向一个固定的心形点云。你可以根据需求调整颜色、速度、旋转等参数。
相关推荐
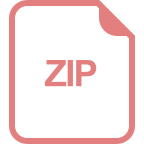
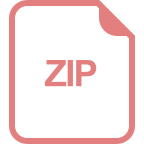
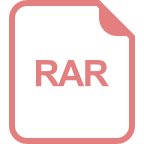














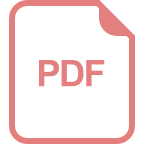