python 用base64解码
时间: 2024-09-13 15:01:31 浏览: 49
Python的base64模块提供了编解码base64字符串的功能,你可以使用它来对数据进行base64编码和解码。解码一个base64字符串很简单,你只需要使用`base64.b64decode()`函数。这个函数接受一个base64编码的字符串作为输入,并返回一个字节串。
以下是一个使用Python的base64模块进行解码的例子:
```python
import base64
# base64编码的字符串
encoded_str = "SGVsbG8gV29ybGQh" # "Hello World!"的base64编码形式
# 对字符串进行解码
decoded_bytes = base64.b64decode(encoded_str)
# 将解码后的字节串转换为字符串
decoded_str = decoded_bytes.decode('utf-8')
print("解码后的字符串:", decoded_str)
```
在这个例子中,我们首先导入了`base64`模块,然后对一个base64编码的字符串进行了解码,并将解码后的字节串转换为UTF-8编码的字符串输出。
相关问题
python 实现base64解码
### 回答1:
可以使用Python的base64模块来实现base64解码,例如:import base64 encoded_data = "YmFzZTY0IGVuY29kZWQgc3RyaW5n" decoded_data = base64.b64decode(encoded_data) print(decoded_data)
### 回答2:
在Python中,我们可以使用内置的base64模块来实现Base64解码。首先,我们需要导入base64模块:
import base64
然后,我们可以使用base64模块的decodebytes()函数来对Base64编码的字符串进行解码。该函数接受一个字符串作为参数,并将其解码为原始的二进制数据。下面是一个示例:
# Base64编码的字符串
encoded_string = "SGVsbG8gV29ybGQh"
# 解码为二进制数据
decoded_bytes = base64.decodebytes(encoded_string.encode('utf-8'))
最后,我们可以将解码后的二进制数据转换为字符串形式,以便于查看和处理。可以使用decode()方法将二进制数据转换为字符串。下面是一个示例:
# 二进制数据转换为字符串
decoded_string = decoded_bytes.decode('utf-8')
现在,我们可以打印解码后的字符串,以验证解码是否成功:
print(decoded_string)
输出:
Hello World!
这样,我们就成功地使用Python实现了Base64解码。
### 回答3:
要实现Python中的Base64解码,可以使用标准库中的base64模块。下面是一个简单的示例:
```Python
import base64
def base64_decode(input_string):
# 将输入字符串转换为bytes类型
input_bytes = input_string.encode('utf-8')
# 使用base64模块的decodebytes函数解码
decoded_bytes = base64.decodebytes(input_bytes)
# 将解码后的bytes转换为字符串并返回
decoded_string = decoded_bytes.decode('utf-8')
return decoded_string
# 测试
encoded_string = 'SGVsbG8gd29ybGQ='
decoded_string = base64_decode(encoded_string)
print(decoded_string)
```
在这个示例中,我们定义了一个名为`base64_decode`的函数,它接受一个字符串作为输入,并返回解码后的字符串。首先,我们将输入字符串转换为bytes类型,然后使用`base64`模块的`decodebytes`函数对其进行解码。最后,我们将解码后的bytes转换为字符串,并返回结果。
在测试部分,我们将一个经过Base64编码的字符串传递给`base64_decode`函数进行解码,然后打印解码后的字符串。输出为`Hello world`,表示解码成功。
python实现base64解码
Python中可以使用`base64`模块来实现Base64解码。以下是一个简单的示例:
```python
import base64
# 需要解码的Base64字符串
encoded_str = "aGVsbG8gd29ybGQ="
# 使用base64模块进行解码
decoded_bytes = base64.b64decode(encoded_str)
# 将解码后的字节转换为字符串
decoded_str = decoded_bytes.decode('utf-8')
print("解码后的字符串:", decoded_str)
```
在这个示例中,我们首先导入了`base64`模块。然后,我们定义了一个需要解码的Base64字符串`encoded_str`。接下来,我们使用`base64.b64decode()`函数对字符串进行解码,得到解码后的字节。最后,我们将解码后的字节转换为字符串并打印出来。
阅读全文
相关推荐
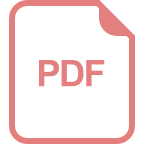
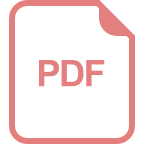
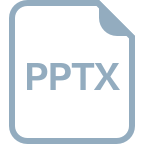













