c语言建立一有序的链表并实现线性表的基本操作(初始化、插入、删除、查找等)。
时间: 2024-10-09 17:01:35 浏览: 57
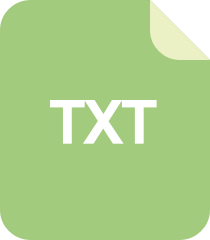
线性表的实现_代码(初始化、判断是否为空表、求表长、输出表、插入、删除、查找、修改、清空表、释放表空间、退出

在C语言中,创建一个有序链表并实现基本操作通常涉及以下几个步骤:
1. **结构体定义**:
首先定义一个节点结构体,包含数据域(data)和指向下一个节点的指针(next)。
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. **初始化**:
初始化函数可以创建一个空链表,链表头指针设置为NULL。
```c
Node* createList() {
return NULL;
}
```
3. **插入元素**:
插入函数需要比较新元素的值,将其插入到正确的位置保持链表有序。
```c
void insert(Node** head, int value) {
if (*head == NULL || (*head)->data > value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL && current->next->data < value) {
current = current->next;
}
newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
}
}
```
4. **删除元素**:
删除操作需要找到要删除的节点,并更新前一个节点的next指针。
```c
void delete(Node** head, int value) {
if (*head != NULL && (*head)->data == value) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
Node* temp = current->next;
current->next = temp->next;
free(temp);
}
}
```
5. **查找元素**:
查找函数遍历链表直到找到匹配项或到达链表尾部。
```c
Node* search(Node* head, int value) {
Node* current = head;
while (current != NULL && current->data != value) {
current = current->next;
}
return current;
}
```
阅读全文
相关推荐
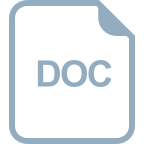
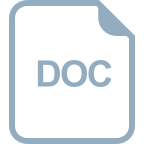

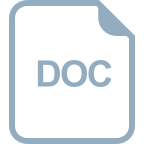








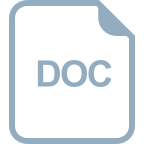
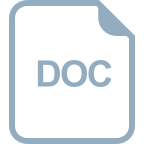


