用c语言编写程序∶顺序线性表:建立,初始化,查找,插入,删除
时间: 2024-10-08 13:22:26 浏览: 39
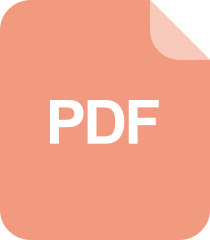
408数据结构.pdf
在C语言中,顺序线性表是一种基础的数据结构,它将数据元素按照一定的顺序存储在一维数组中。下面是一些基本操作的概述:
**1. 建立(创建)顺序线性表**
首先,你需要定义一个结构体或共用体来表示表中的每个元素,并动态分配一维数组来存储这些元素。例如:
```c
typedef struct {
int data;
struct Node* next; // 如果是链式表,则需要next指针
} Node;
// 创建链表
Node* createList(int capacity) {
Node* list = (Node*)malloc(capacity * sizeof(Node));
list[0].next = NULL;
return list;
}
```
**2. 初始化(填充)顺序线性表**
对数组中的所有元素赋初值,如果是链表则可以为空或设置默认值:
```c
void initialize(Node* list, int values[], int size) {
for (int i = 0; i < size; i++) {
list[i].data = values[i];
}
}
```
**3. 查找(搜索)**
查找特定值的位置或是否存在,遍历数组或链表:
```c
int search(Node* list, int value) {
for (int i = 0; list[i].data != value && list[i].next != NULL; i++) {
// 遍历元素
}
if (list[i].data == value) {
return i; // 找到返回索引
} else {
return -1; // 未找到
}
}
```
**4. 插入(添加)**
在指定位置插入新的元素:
- 数组中插入:移动后面的元素并更新指针;
- 链表中插入:新建节点,指向目标节点,然后调整前驱节点的next指针。
```c
void insert(Node** list, int index, int value) {
// 数组插入示例
if (index >= 0 && index < list->size) {
memmove(&list[index + 1], &list[index], (list->size - index) * sizeof(*list));
list[index].data = value;
}
// 链表插入示例
else {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = list[index];
list[index] = newNode;
}
}
```
**5. 删除(移除)**
删除指定位置或特定值的元素:
- 数组中删除:直接覆盖元素;
- 链表中删除:更新前驱节点的next指针。
```c
void delete(Node** list, int index, int value) {
if (index < 0 || index >= list->size) return;
// 数组删除示例
if (list[index].data == value) {
memmove(list + index, list + index + 1, (list->size - index - 1) * sizeof(*list));
}
// 链表删除示例
else if (list[index]->data == value) {
Node* temp = list[index];
list[index] = list[index]->next;
free(temp);
}
}
```
阅读全文
相关推荐
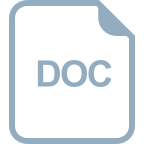
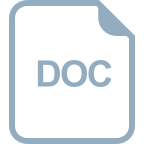
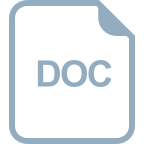
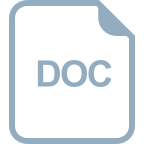
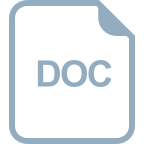
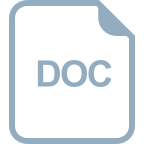
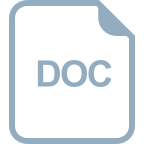
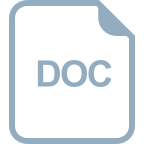
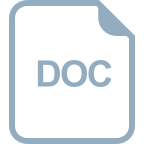







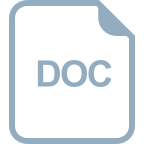
