用c语言编写一个程序实现如下功能,水平类比大二学生Q1 实现线性表的顺序存储结构,并利用文件中的内容初始化Q2 实现线性表的顺序输出、获取长度、查找、插入、删除Q3 删除所有score低于s的元素 Q4 将线性表内容按顺序输出到文件
时间: 2024-09-26 07:16:50 浏览: 45
在C语言中,我们可以按照以下步骤创建一个简单的线性表结构,包括顺序存储、文件操作以及基本操作:
1. **定义线性表结构**:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 初始化线性表
void initializeList(Node** head, FILE* file) {
if (!file) {
printf("Error: File not found.\n");
return;
}
int data;
while (fscanf(file, "%d", &data) == 1) {
*head = createNode(data);
(*head)->next = *head + 1; // 这里假设数据按顺序连续读取
head = &(*head)->next;
}
}
```
2. **线性表操作函数**:
- 获取长度:通过遍历链表计算元素数
- 查找:遍历链表寻找特定值
- 插入:在指定位置插入新节点
- 删除:找到并移除特定节点
- 输出到文件:将链表内容写回文件
```c
int getLength(Node* head) {
int count = 0;
Node* temp = head;
while (temp != NULL) {
count++;
temp = temp->next;
}
return count;
}
// 更改这里的search和delete函数来实现查找和删除操作
int search(Node* head, int value) { ... }
void insert(Node** head, int data, int index) {
Node* newNode = createNode(data);
if (index == 0) {
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
for (int i = 0; i < index - 1 && current != NULL; i++) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
}
void deleteElement(Node** head, int value) {
// 在这里实现删除操作,比如使用双向链表会方便一些
}
void outputToFile(Node* head, FILE* file) {
int length = getLength(head);
fprintf(file, "Output:\n");
for (int i = 0; i < length; i++) {
fprintf(file, "%d ", head->data);
head = head->next;
}
fclose(file);
}
```
3. **主函数**:
```c
int main() {
FILE* file = fopen("input.txt", "r"); // 打开文件
if (file == NULL) {
printf("Failed to open the file.\n");
return 1;
}
Node* head = NULL;
initializeList(&head, file);
// 使用初始化后的列表,进行其他操作
...
fclose(file); // 关闭文件
return 0;
}
```
阅读全文
相关推荐
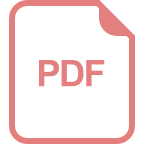
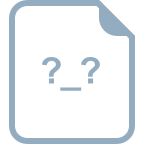
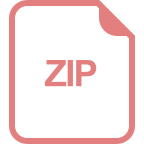















