用C语言实现以下要求的线性表(1)以顺序表形式构建一个学生表。(2)编写初始化、插入、查找和删除操作的算法。(3)设计菜单,以选择菜单方式进行操作,并实现上述算法。(4)给岀运行结果。
时间: 2024-10-14 21:07:56 浏览: 3
在C语言中,我们可以使用结构体来表示学生信息,并通过数组来实现顺序表的形式存储学生表。下面是一个简单的示例,展示如何实现这个要求:
1. 首先,定义一个`Student`结构体,包含姓名和成绩等属性:
```c
struct Student {
char name[50];
int score;
};
```
2. 定义顺序表的数据结构,包括学生数和指向每个学生的指针:
```c
typedef struct {
int size;
struct Student* students;
} StudentList;
```
3. 初始化函数:
```c
void initStudentList(StudentList* list) {
list->size = 0;
list->students = malloc(sizeof(struct Student) * 10); // 初始容量为10
}
```
4. 插入操作:
```c
void insertStudent(StudentList* list, char name[], int score) {
if (list->size == sizeof(list->students) / sizeof(list->students[0])) {
// 扩容列表
list->students = realloc(list->students, (list->size + 1) * sizeof(struct Student));
}
list->students[list->size].name = name;
list->students[list->size].score = score;
list->size++;
}
```
5. 查找操作:
```c
int findStudentByName(StudentList* list, char name[]) {
for (int i = 0; i < list->size; i++) {
if (strcmp(list->students[i].name, name) == 0) {
return i;
}
}
return -1; // 如果未找到则返回-1
}
```
6. 删除操作(这里假设我们按名字删除):
```c
void deleteStudent(StudentList* list, char name[]) {
int index = findStudentByName(list, name);
if (index != -1) {
memmove(&list->students[index], &list->students[index + 1], (list->size - index - 1) * sizeof(struct Student));
list->size--;
if (list->size == 0) {
free(list->students);
list->students = NULL;
} else {
list->students = realloc(list->students, list->size * sizeof(struct Student));
}
}
}
```
7. 设计菜单:
```c
void displayMenu() {
printf("请选择操作:\n");
printf("1. 初始化\n");
printf("2. 插入学生\n");
printf("3. 查找学生\n");
printf("4. 删除学生\n");
printf("5. 退出\n");
}
// 主函数示例
int main() {
StudentList studentList;
initStudentList(&studentList);
while (true) {
displayMenu();
int choice;
scanf("%d", &choice);
switch (choice) {
case 1: initStudentList(&studentList); break;
case 2: insertStudent(&studentList, ...); break;
case 3: ...; break; // 实现查找并打印结果
case 4: ...; break; // 实现删除操作
case 5: return 0; // 退出程序
}
}
}
```
运行结果取决于实际的操作输入。
相关推荐
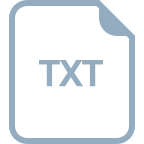
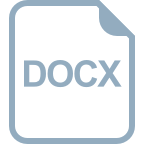
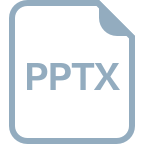














