如何使用Java编程语言通过FTP来实现文件的上传、下载、查找和删除功能?
时间: 2024-11-05 21:33:11 浏览: 7
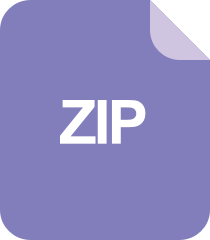
java FTP服务器文件上传下载,创建多级文件夹.zip
使用Java编程语言通过FTP来实现文件的上传、下载、查找和删除功能,通常会依赖于像Apache Commons Net这样的FTP库。这里是一个简要的示例:
### 1. **文件上传(PUT):**
```java
import org.apache.commons.net.ftp.FTPClient;
public void uploadFile(String server, String user, String pass, String filePath, String remotePath) throws IOException {
FTPClient client = new FTPClient();
client.connect(server);
client.login(user, pass);
client.changeWorkingDirectory(remotePath); // 如果需要先切换到目标目录
FileInputStream fis = new FileInputStream(filePath);
boolean result = client.storeFile(new File(remotePath).getName(), fis);
if (result) {
System.out.println("文件 " + filePath + " 上传成功");
} else {
System.out.println("文件上传失败");
}
client.logout();
client.disconnect();
}
```
### 2. **文件下载(GET):**
```java
import org.apache.commons.net.ftp.FTPClient;
public void downloadFile(String server, String user, String pass, String remotePath, String localPath) throws IOException {
FTPClient client = new FTPClient();
client.connect(server);
client.login(user, pass);
client.changeWorkingDirectory("/"); // 如果需要先回到根目录
InputStream fis = client.retrieveFileStream(remotePath);
FileOutputStream fos = new FileOutputStream(localPath);
byte[] buffer = new byte[1024];
while (true) {
int bytesRead = fis.read(buffer);
if (bytesRead == -1) break; // 文件读取完成
fos.write(buffer, 0, bytesRead);
}
fis.close();
fos.close();
System.out.println("文件 " + remotePath + " 下载到本地 " + localPath);
client.logout();
client.disconnect();
}
```
### 3. **文件查找(LIST):**
```java
import org.apache.commons.net.ftp.FTPClient;
public void listFiles(String server, String user, String pass) throws IOException {
FTPClient client = new FTPClient();
client.connect(server);
client.login(user, pass);
String[] files = client.listFiles("/");
for (String fileName : files) {
System.out.println(fileName);
}
client.logout();
client.disconnect();
}
```
### 4. **文件删除(DELETE):**
```java
import org.apache.commons.net.ftp.FTPClient;
public void deleteFile(String server, String user, String pass, String remotePath) throws IOException {
FTPClient client = new FTPClient();
client.connect(server);
client.login(user, pass);
boolean success = client.deleteFile(remotePath);
if (success) {
System.out.println("文件 " + remotePath + " 删除成功");
} else {
System.out.println("文件删除失败");
}
client.logout();
client.disconnect();
}
```
以上代码需注意异常处理,并根据实际需求进行适当调整。
阅读全文
相关推荐
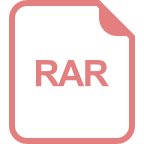
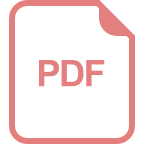
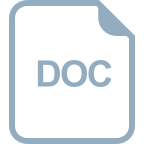
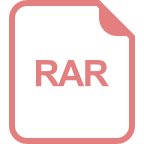
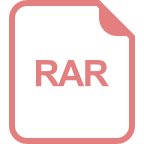
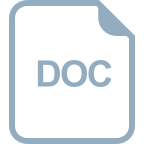
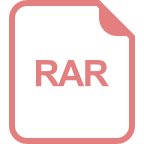
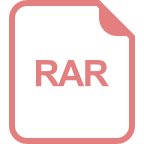
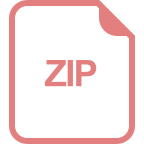
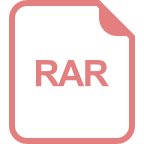
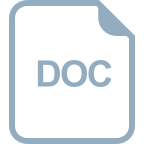
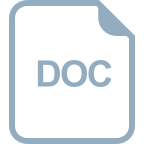
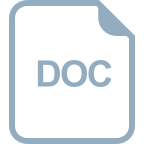
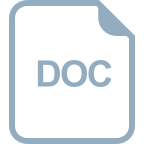
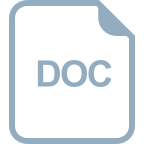
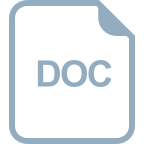
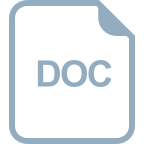
