c++ content-type
时间: 2023-11-03 17:53:40 浏览: 161
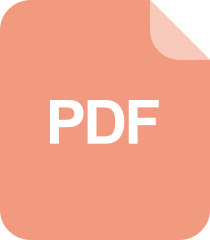
http中content-type头值-1
在C语言中,要实现不同content-type的POST请求,你可以使用C库中的CGI(公共网关接口)来处理。CGI库提供了处理HTTP请求和响应的功能。你可以通过设置HTTP头部中的content-type字段来指定不同的content-type。
以下是一个示例代码,展示了如何在C语言中实现发送POST请求并设置不同content-type的方法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <curl/curl.h>
int main(void) {
CURL *curl;
CURLcode res;
// 初始化CURL库
curl_global_init(CURL_GLOBAL_ALL);
// 创建CURL句柄
curl = curl_easy_init();
if(curl) {
// 设置请求的URL
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
// 设置POST请求
curl_easy_setopt(curl, CURLOPT_POST, 1L);
// 设置请求的内容和长度
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "content=example");
curl_easy_setopt(curl, CURLOPT_POSTFIELDSIZE, strlen("content=example"));
// 设置content-type为application/x-www-form-urlencoded
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "Content-Type: application/x-www-form-urlencoded");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
// 发送请求
res = curl_easy_perform(curl);
// 清理
curl_easy_cleanup(curl);
}
// 清理CURL库
curl_global_cleanup();
return 0;
}
```
在上面的示例代码中,我们使用了libcurl库来发送HTTP请求。通过调用curl_easy_setopt函数,我们可以设置POST请求、请求的URL、请求的内容和长度以及请求的content-type。在这个例子中,我们设置了content-type为application/x-www-form-urlencoded。
这只是一个示例,你可以根据你的需求设置不同的content-type,例如application/json、multipart/form-data等。只需根据需要设置HTTP头部中的content-type字段即可。
提供了一种封装好的方式,你可以直接使用它来发送不同content-type的POST请求。
阅读全文
相关推荐
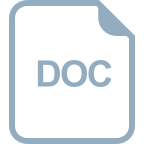
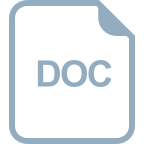






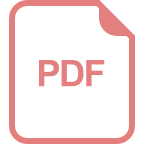



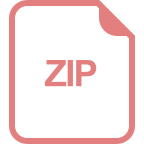
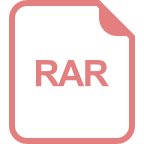


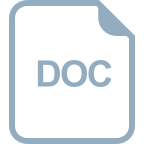
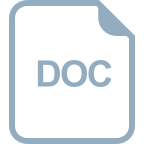