用yolov5实时识别并输出位置数据代码
时间: 2024-09-21 09:11:36 浏览: 49
YOLOv5(You Only Look Once version 5)是一个流行的基于深度学习的目标检测算法,它可以在视频流中实现实时目标检测。以下是使用Python和PyTorch实现YOLOv5实时识别并输出位置数据的基本步骤:
```python
import torch
from PIL import Image
from torchvision.transforms import functional as F
from yolov5.models.experimental import attempt_load
# 加载预训练模型
model = attempt_load('yolov5s', map_location='cuda') if torch.cuda.is_available() else attempt_load('yolov5s', map_location=torch.device('cpu'))
# 定义处理输入图像的函数
def preprocess_image(image_path):
img = Image.open(image_path)
img = F.to_tensor(img)
img = img.unsqueeze(0) # 添加batch dimension
return img
# 实时识别并输出位置数据
def detect_objects(image_path):
img = preprocess_image(image_path)
results = model(img) # 检测结果
boxes, labels, scores = results.xyxy[0], results.labels[0], results.confidences[0]
for box, label, score in zip(boxes, labels, scores):
x1, y1, x2, y2 = box
position_data = {'label': label.item(), 'score': round(score.item(), 2), 'top_left': (x1, y1), 'bottom_right': (x2, y2)}
print(position_data)
# 使用你的图片路径替换下面这行
detect_objects('path_to_your_image.jpg')
```
这个示例假设你已经下载了YOLOv5的预训练模型,并将其放在`yolov5s.pt`文件下。运行此代码时,它会读取指定图像,进行预测,然后打印出每个检测到物体的位置、标签和置信度。
阅读全文
相关推荐
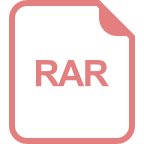
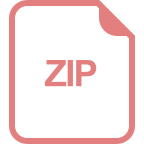
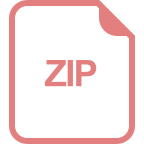
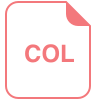






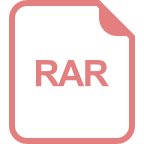
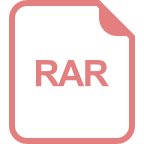
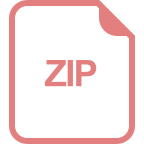
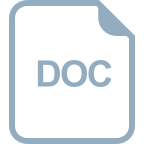
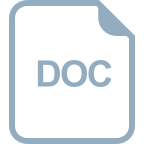
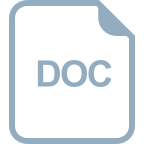
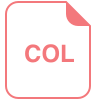
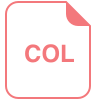
