python requests 发送短信
时间: 2023-11-06 12:02:26 浏览: 61
使用Python的requests库可以发送HTTP请求,包括发送短信。你可以使用requests库中的get或post方法来发送请求,并通过指定URL和请求头来实现发送短信的功能。例如,你可以使用以下代码来发送请求并获取响应:
```python
import requests
# 设置请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 发送GET请求
response = requests.get('https://app.fzxtech.com/gs-api/register/sendcode2', headers=headers)
# 获取响应内容
print(response.text)
```
需要注意的是,你可能需要根据具体的API接口来调整请求的URL、请求头和请求方法。此外,你还可以使用requests库的其他方法和参数来进一步控制请求,例如设置请求参数、发送POST请求等。
相关问题
python向手机发送短信
你可以使用以下代码实现Python向手机发送短信的功能:
```python
import requests
class SMS:
"""短信发送功能"""
def __init__(self, account, password):
"""account: APIID(用户中心【验证码通知短信】-【产品纵览】查看)
password:APIKEY(用户中心【验证码通知短信】-【产品纵览】查看)
self.url: 接口请求地址
"""
self.account = account
self.password = password
self.url = 'https://106.ihuyi.com/webservice/sms.php?method=Submit'
def send_sms(self, mobile, content):
""" 发短信
:param mobile: 手机号
:param content: 短信内容
:return: None
"""
headers = {
"Content-type": "application/x-www-form-urlencoded",
"Accept": "text/plain"
}
data = {
'account': self.account,
'password': self.password,
'mobile': mobile,
'content': content
}
# 发起请求
response = requests.post(self.url, headers=headers, data=data)
print(response.content.decode())
if __name__ == '__main__':
sms = SMS('C4xxxxx41', '46c4xaxxxxxxxxxxxxcd4')
sms.send_sms('157xxxx1995', '您的验证码是:8888。请不要把验证码泄露给其他人。')
```
python 收取手机短信
使用Python收取手机短信通常需要借助第三方工具或服务。下面将介绍一种常见的方法。
首先,你需要准备一个运行Python代码的环境,比如安装Python解释器和相应的开发包。
其次,你需要选择一个合适的短信接口和服务商。一些知名的短信接口服务提供商有云片网、容联云通讯等。你可以在他们的官方网站上注册账号,并获取相关的API密钥。
然后,你需要使用python的请求库(比如requests)来发送HTTP请求。根据短信接口文档的要求,以POST方式发送请求,并提供合适的参数,比如手机号码、短信内容等。
最后,你可以使用Python的json库解析短信接口返回的结果。通常,短信接口会返回一个JSON格式的响应,其中包含了发送短信的状态、错误码等信息。
需要注意的是,由于涉及到短信安全和隐私等问题,国内的短信接口服务商对短信的发送进行了严格的管控。在使用之前,你可能需要进行身份验证或者符合相关的政策要求。
总之,使用Python收取手机短信涉及到选择合适的短信接口服务商、发送HTTP请求,并解析响应结果的过程。具体的实现方式和细节取决于你选择的短信接口和服务商。
相关推荐
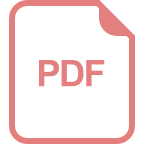
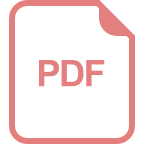












