数据结构顺序串的基本操作C语言
时间: 2023-11-12 11:08:19 浏览: 147
顺序串是一种线性表的存储结构,它是由一组连续的存储单元来存储的。在C语言中,我们可以使用结构体来定义顺序串,如下所示:
```
#define MAXSIZE 256
typedef struct String {
char ch[MAXSIZE];
int length;
} String;
```
其中,`ch`数组用来存储串的每个元素,`length`表示串的长度。下面是顺序串的基本操作:
1. 初始化串
```
void InitString(String* s) {
s->ch[0] = '\0';
s->length = 0;
}
```
2. 串赋值
```
void StrAssign(String* s, char* str) {
int i = 0;
while (str[i] != '\0') {
s->ch[i] = str[i];
i++;
}
s->ch[i] = '\0';
s->length = i;
}
```
3. 串拼接
```
void StrConcat(String* s1, String* s2) {
int i = 0;
while (s2->ch[i] != '\0') {
s1->ch[s1->length + i] = s2->ch[i];
i++;
}
s1->ch[s1->length + i] = '\0';
s1->length += i;
}
```
4. 串比较
```
int StrCompare(String* s1, String* s2) {
int i = 0;
while (s1->ch[i] != '\0' && s2->ch[i] != '\0') {
if (s1->ch[i] != s2->ch[i]) {
return s1->ch[i] - s2->ch[i];
}
i++;
}
return s1->length - s2->length;
}
```
5. 串复制
```
void StrCopy(String* s1, String* s2) {
int i = 0;
while (s2->ch[i] != '\0') {
s1->ch[i] = s2->ch[i];
i++;
}
s1->ch[i] = '\0';
s1->length = s2->length;
}
```
阅读全文
相关推荐
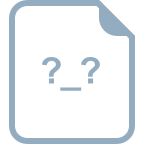
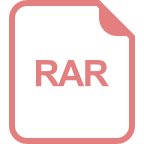















