用Java语言实现在D盘中创建文件test.txt,文件中内容为“hello Java"然后利用流把该文件拷贝到E盘根目录下。
时间: 2024-11-22 17:39:16 浏览: 15
在Java中,你可以使用`java.io.File`类和`java.nio.file.Files`类来实现这个操作。首先,我们需要获取D盘和E盘的路径,然后创建文件并写入内容,最后将文件复制到目标位置。以下是一个示例:
```java
import java.io.*;
import java.nio.file.*;
public class FileCopyExample {
public static void main(String[] args) {
String dDrivePath = "D:\\";
String filePath = dDrivePath + "test.txt";
String eDrivePath = "E:\\";
try {
// 创建文件并写入内容
Files.write(Paths.get(filePath), ("hello Java".getBytes()));
// 检查目标路径是否已存在,不存在则创建
Path targetPath = Paths.get(eDrivePath);
if (!Files.exists(targetPath)) {
Files.createDirectories(targetPath);
}
// 将文件复制到E盘根目录
Files.copy(Paths.get(filePath), Paths.get(eDrivePath + "test.txt"));
System.out.println("File copied successfully.");
} catch (IOException e) {
System.err.println("An error occurred while copying the file: " + e.getMessage());
}
}
}
```
在这个例子中,我们使用了`Files.write()`方法创建文件并写入文本,以及`Files.copy()`方法来复制文件。
阅读全文
相关推荐
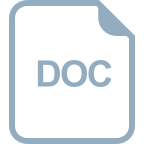
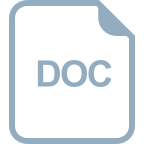
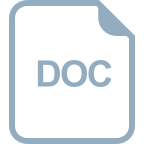
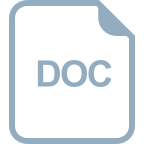
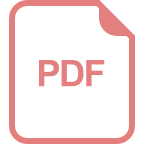
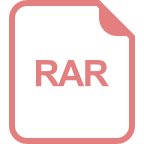
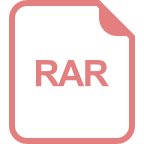
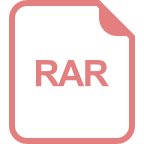
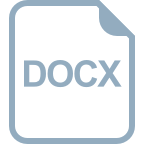
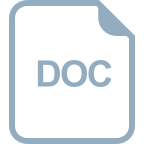
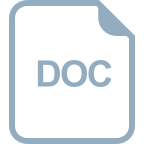
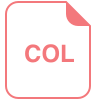
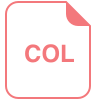
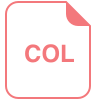
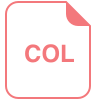
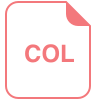
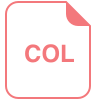
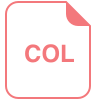