C代码实现PWM 蜡烛灯效果
时间: 2024-12-03 19:31:27 浏览: 10
在C语言中,为了实现PWM控制蜡烛灯效果,我们需要模拟一个周期性的亮度变化。假设我们有一个GPIO引脚连接到LED灯,并且通过PWM来调节其亮度。这里是一个基本的示例,使用Linux的`sysfs` API来访问GPIO和PWM,如Pulse Width Modulation (PWM)功能通常在许多微控制器上可用。
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <linux/pwm.h>
#include <stdlib.h>
#define PWM_CHANNEL 0 // PWM通道号,通常从0开始计数
#define LED_GPIO_PIN 18 // LED灯对应的GPIO引脚
int main() {
int fd, pwm_fd;
struct pwm_device_info devinfo;
struct pwm_config config;
struct pwm_range range;
// 打开sysfs文件系统
if ((fd = open("/dev/pwmchip" PWM_CHANNEL, O_RDWR)) < 0) {
perror("Failed to open /dev/pwmchip");
return -1;
}
// 获取PWM设备信息
if (ioctl(fd, PWM_GET_INFO, &devinfo) < 0) {
perror("Failed to get PWM device info");
close(fd);
return -1;
}
// 配置PWM频率
config.freq = 50; // 设置PWM频率,比如50Hz
config.duty_cycle_min = 0; // 最小占空比
config.duty_cycle_max = 100; // 最大占空比,对应100%亮度
// 检查是否支持范围设置
if (ioctl(fd, PWM_SET_RANGE, &range) == 0) {
printf("Setting PWM range.\n");
range.period = devinfo.max_period; // 使用最大周期
} else {
printf("Range setting not supported.\n");
}
// 创建PWM实例
if ((pwm_fd = open(devinfo.name, O_WRONLY | O_NONBLOCK)) < 0) {
perror("Failed to create PWM instance");
close(fd);
return -1;
}
// 开始PWM并设置初始占空比(0%亮度)
if (ioctl(pwm_fd, PWM_ENABLE, 0) < 0) {
perror("Failed to enable PWM");
}
if (write(pwm_fd, &config, sizeof(config)) < 0) {
perror("Failed to set PWM configuration");
}
for (int i = 0; i <= 100; i++) { // 循环改变占空比,模拟从熄灭到点亮的过程
uint16_t duty_cycle = i * devinfo.max_period / 100; // 计算当前亮度
write(pwm_fd, &duty_cycle, sizeof(duty_cycle)); // 更新占空比
usleep(10000); // 等待一段时间展示闪烁效果
}
// 关闭PWM
if (ioctl(pwm_fd, PWM_DISABLE, 0) < 0) {
perror("Failed to disable PWM");
}
close(pwm_fd);
close(fd);
return 0;
}
阅读全文
相关推荐
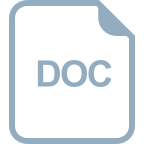
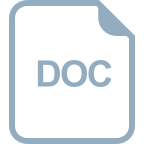
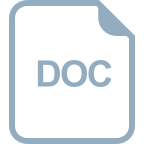
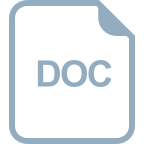
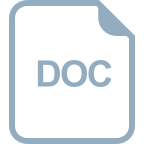
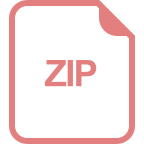
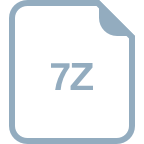
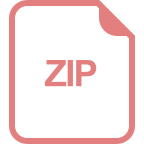
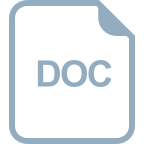
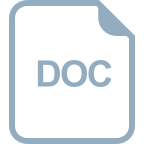
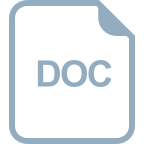
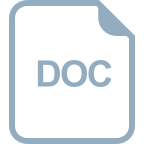
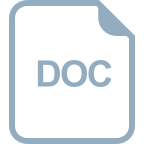
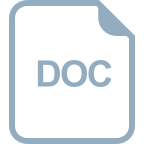
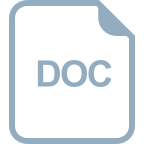
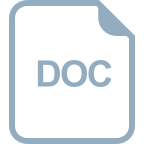

