c++如何主动抛出错误
时间: 2024-09-06 14:04:23 浏览: 59
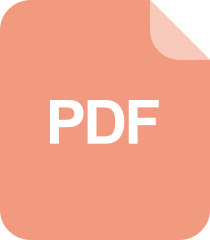
C与C++中的异常处理.pdf
在C++中,主动抛出错误通常使用`throw`关键字配合自定义或标准异常类型来完成。当你认为某个情况不应该正常执行,而是应该立即终止函数的执行流程,并让调用者处理这个问题时,你可以这样做。以下是一个简单的例子:
```cpp
#include <iostream>
#include <stdexcept>
// 自定义异常类型
class CustomException : public std::runtime_error {
public:
CustomException(const std::string& message) : std::runtime_error(message) {}
};
void doSomethingThatCouldThrow(int x) {
if (x < 0) {
// 当条件不符合预期,主动抛出错误
throw CustomException("Invalid argument: x must be non-negative");
}
// 正常操作...
}
int main() {
try {
doSomethingThatCouldThrow(-5);
} catch (const CustomException& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
在这个示例中,`doSomethingThatCouldThrow`函数检查`x`是否非负。如果`x`小于零,它会主动抛出一个自定义的`CustomException`异常,并传递一个错误消息。在`main`函数中,我们用`try-catch`结构捕获并处理可能抛出的异常。
阅读全文
相关推荐
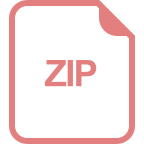
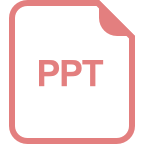
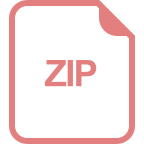
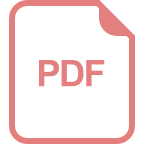
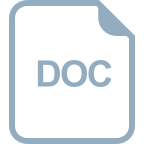
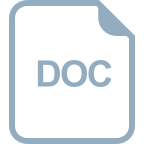
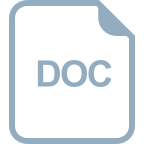
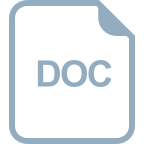
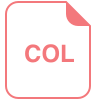
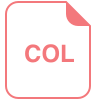
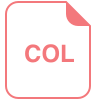
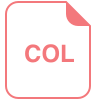
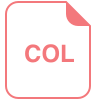

