BeanUtils.copyProperties忽略null值
时间: 2023-11-21 15:55:56 浏览: 144
可以使用BeanMapper或者在BeanUtils.copyProperties中传入一个自定义的属性过滤器来忽略null值。以下是两种方法的示例代码:
1. 使用BeanMapper忽略null值
```python
import net.sf.cglib.beans.BeanCopier;
import net.sf.cglib.core.Converter;
public class BeanMapper {
private static final Map<String, BeanCopier> BEAN_COPIERS = new ConcurrentHashMap<>();
public static void copyProperties(Object source, Object target) {
String key = source.getClass().toString() + target.getClass().toString();
BeanCopier copier = null;
if (!BEAN_COPIERS.containsKey(key)) {
copier = BeanCopier.create(source.getClass(), target.getClass(), true);
BEAN_COPIERS.put(key, copier);
} else {
copier = BEAN_COPIERS.get(key);
}
copier.copy(source, target, new Converter() {
@Override
public Object convert(Object value, Class target, Object context) {
if (value == null) {
if (target == String.class) {
return "";
} else if (target == Integer.class) {
return 0;
} else if (target == Long.class) {
return 0L;
} else if (target == Double.class) {
return 0.0;
} else if (target == Float.class) {
return 0.0f;
} else if (target == Boolean.class) {
return false;
} else {
return null;
}
} else {
return value;
}
}
});
}
}
```
使用示例:
```python
Source source = new Source();
source.setName("test");
source.setAge(18);
source.setAddress(null);
Target target = new Target();
BeanMapper.copyProperties(source, target);
System.out.println(target.getName()); // 输出:test
System.out.println(target.getAge()); // 输出:18
System.out.println(target.getAddress()); // 输出:null
```
2. 在BeanUtils.copyProperties中传入自定义属性过滤器忽略null值
```python
import org.springframework.beans.BeanUtils;
import org.springframework.beans.BeanWrapper;
import org.springframework.beans.BeanWrapperImpl;
import java.beans.PropertyDescriptor;
import java.util.HashSet;
import java.util.Set;
public class CopyUtils {
public static void copyPropertiesIgnoreNull(Object source, Object target) {
BeanUtils.copyProperties(source, target, getNullPropertyNames(source));
}
private static String[] getNullPropertyNames(Object source) {
final BeanWrapper src = new BeanWrapperImpl(source);
PropertyDescriptor[] pds = src.getPropertyDescriptors();
Set<String> emptyNames = new HashSet<>();
for (PropertyDescriptor pd : pds) {
Object srcValue = src.getPropertyValue(pd.getName());
if (srcValue == null) {
emptyNames.add(pd.getName());
}
}
String[] result = new String[emptyNames.size()];
return emptyNames.toArray(result);
}
}
```
使用示例:
```python
Source source = new Source();
source.setName("test");
source.setAge(18);
source.setAddress(null);
Target target = new Target();
CopyUtils.copyPropertiesIgnoreNull(source, target);
System.out.println(target.getName()); // 输出:test
System.out.println(target.getAge()); // 输出:18
System.out.println(target.getAddress()); // 输出:null
```
阅读全文
相关推荐
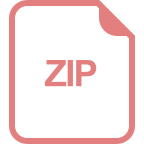
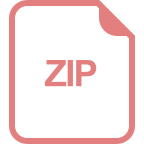
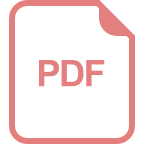















