html获取的数据填入oracle代码
时间: 2024-10-14 10:17:09 浏览: 21
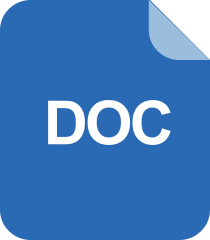
JAVA实现Excel表数据导入ORACLE数据库

在HTML页面上获取数据通常通过JavaScript或其他前端技术如AJAX完成,然后将这些数据传递给服务器端。如果你打算将这些数据插入到Oracle数据库中,你需要在服务器端编程语言(比如Java、Python、PHP等)中处理这部分逻辑。
以下是一个简单的示例,使用Java(Spring Boot + JDBC):
1. **HTML(前端)**:
```html
<!DOCTYPE html>
<html>
<body>
<button onclick="sendData()">提交数据</button>
<script>
function sendData() {
var name = document.getElementById("name").value;
var age = document.getElementById("age").value;
// 发送Ajax请求
$.ajax({
url: '/insert-data',
type: 'POST',
data: { name: name, age: age },
success: function(response) {
console.log('数据已成功插入');
},
error: function(err) {
console.error('数据插入失败', err);
}
});
}
</script>
...
</body>
</html>
```
2. **Java (后端 Spring Controller)**:
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class DataController {
@PostMapping("/insert-data")
public String insertData(@RequestBody InsertRequest insertRequest) {
// 获取HTML表单传来的数据
String name = insertRequest.getName();
int age = insertRequest.getAge();
try {
// 连接到Oracle数据库并执行SQL插入
String sql = "INSERT INTO your_table (name, age) VALUES (:name, :age)";
jdbcTemplate.update(sql, new Object[]{name, age});
return "数据插入成功";
} catch (Exception e) {
throw new RuntimeException("数据插入失败", e);
}
}
// 数据模型类
static class InsertRequest {
private String name;
private int age;
// getters and setters
}
}
```
在这个例子中,前端发送的`name`和`age`数据会通过Ajax请求到达`/insert-data`路径,后端Java代码接收到数据后,将其插入到指定的Oracle表中。
阅读全文
相关推荐
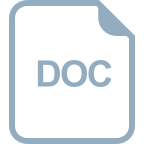
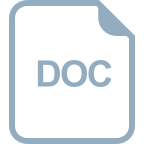
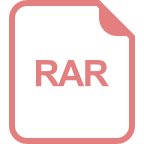
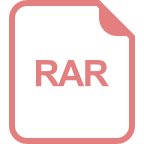
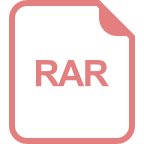
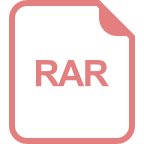
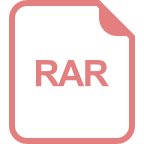
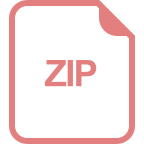
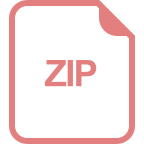
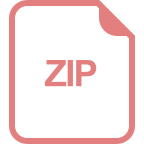
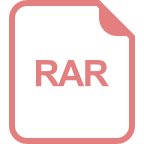
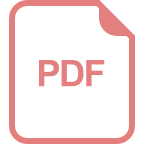
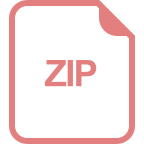
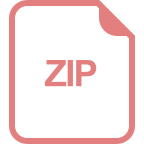
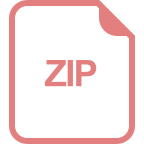
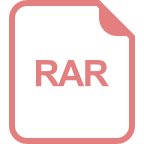