PHP 怎么判断当前时间是否在 周四至周一 20:00~03:00 的营业时间?
时间: 2024-09-19 13:13:49 浏览: 70
在PHP中,你可以通过以下步骤来判断当前时间是否在周四至周一的晚上8点到凌晨3点之间:
首先,获取当前日期和时间,可以使用`date()`函数,例如 `date('Y-m-d H:i:s')`。
然后,你需要将这个时间拆分为星期几(0代表周日,1代表周一,以此类推),小时,分钟和秒。可以使用`date('w')`得到星期几的数字形式,`date('H:i:s')`获取小时、分钟和秒。
接着,你可以编写一个条件来检查星期数、小时和分钟。如果满足以下条件:
1. 星期四(4)至星期一(1)之间的任意一天;
2. 小时在20(即19:00,因为24小时制)到3(即03:00)之间,包括这两个时间点。
以下是简单的代码示例:
```php
$now = date('Y-m-d H:i:s');
$dayOfWeek = (int)date('w'); // 获取星期,0表示周日,1表示周一...
$hours = (int)date('H'); // 获取小时
// 判断日期和时间
if ($dayOfWeek >= 4 && $dayOfWeek <= 1 && $hours >= 19 && $hours < 4) {
echo "现在的时间在营业时间内";
} else {
echo "现在的时间不在营业时间内";
}
相关问题
php 获取半年内的周一和周四
你可以使用PHP的日期函数来获取半年内的周一和周四。下面是一个示例代码:
```php
// 半年前的时间戳
$six_months_ago = strtotime('-6 months');
// 循环遍历半年内的每一天
for ($i = $six_months_ago; $i <= time(); $i += 86400) {
// 获取当前日期是周几
$day_of_week = date('N', $i);
// 如果当前日期是周一或周四
if ($day_of_week == 1 || $day_of_week == 4) {
// 输出当前日期
echo date('Y-m-d', $i) . "\n";
}
}
```
上面的代码会循环遍历半年内的每一天,然后判断当前日期是周一或周四,如果是则输出当前日期。你可以根据自己的需求修改输出的日期格式。
前端如何对对象数组按属性值进行排序 如[{"周一":2},{"周日"::3},{"周四"::7}]
可以使用数组的`sort()`方法来对对象数组按属性值进行排序。你可以传入一个比较函数作为参数,该函数将比较两个元素的属性值,并根据比较结果返回一个负数、零或正数来决定排序顺序。
下面是一个示例代码,按照对象数组中每个对象的属性值进行排序:
```javascript
const arr = [
{"周一": 2},
{"周日": 3},
{"周四": 7}
];
arr.sort((a, b) => {
const valueA = Object.values(a)[0];
const valueB = Object.values(b)[0];
return valueA - valueB;
});
console.log(arr); // 输出: [{"周一":2},{"周日":3},{"周四":7}]
```
在这个例子中,我们使用了`Object.values()`方法获取对象的属性值,并在比较函数中进行排序。
阅读全文
相关推荐
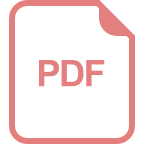
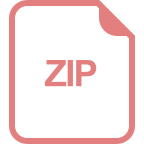
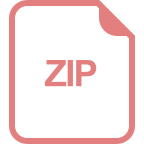













