def convertTemperature(self, celsius: float) -> List[float]:
时间: 2024-10-19 22:13:39 浏览: 121
这个函数 `convertTemperature` 是用于将摄氏温度 (`celsius`) 转换为其他温度单位(如华氏、开尔文等)的。它接受一个浮点数类型的 `celsius` 参数,代表待转换的摄氏温度,并返回一个浮点数列表,包含了转换后的温度值。
在 Python 类的方法中,`self` 指的是该类的对象实例,所以这个函数很可能是在某个表示温度转换类的上下文中定义的。例如,如果你有一个 `TemperatureConverter` 类,那么这个函数可能是其中的一个成员方法。
```python
class TemperatureConverter:
def convertTemperature(self, celsius: float) -> List[float]:
fahrenheit = celsius * 9 / 5 + 32 # 华氏度公式
kelvin = celsius + 273.15 # 开尔文公式
return [fahrenheit, kelvin]
# 使用示例
temp_converter = TemperatureConverter()
temperatures_in_fahrenheit_and_kelvin = temp_converter.convertTemperature(25)
```
相关问题
3. (简答题)For converting temperature from Celsius to Fahrenheit, or from Fahrenheit to Celsius, we can use the following formulas. -------------------------------------------------- Celsius To Fahrenheit: Fahrenheit = ( Celsius * 9 / 5 ) + 32; Fahrenheit To Celsius: Celsius = ( Fahrenheit - 32 ) * 5 / 9; -------------------------------------------------- Write a program to convert temperature from Fahrenheit to Celsius and vice versa using switch case. In the program, there are three options given to the user. The first choice is to convert the temperature from Celsius to Fahrenheit. The second choice is to convert the temperature from Fahrenheit to Celsius. And the last one is to exit without testing any one of the queries. The program should work as follows: ---------------------------------------------------------- Select Code: For Fahrenheit To Celsius For Celsius To Fahrenheit Enter Your Choice: 1 Enter The Value of Fahrenheit Temperature: 90 Celsius Temperature: 32.2 ---------------------------------------------------------- Select Code: 1. For Fahrenheit To Celsius 2. For Celsius To Fahrenheit Enter Your Choice:2 Enter The Value of Celsius Temperature: 30 Fahrenheit Temperature: 86.0 ---------------------------------------------------------- Select Code: 1. For Fahrenheit To Celsius 2. For Celsius To Fahrenheit Enter Your Choice:3 Wrong Choice.....Try Again!!! ----------------------------------------------------------
以下是使用 switch case 实现温度转换的程序:
```c++
#include <iostream>
using namespace std;
int main() {
int choice;
float temp, result;
while (true) {
cout << "Select Code:\n";
cout << "1. For Fahrenheit To Celsius\n";
cout << "2. For Celsius To Fahrenheit\n";
cout << "3. Exit\n";
cout << "Enter Your Choice: ";
cin >> choice;
if (choice == 3) {
cout << "Exiting...";
break;
}
switch (choice) {
case 1:
cout << "Enter The Value of Fahrenheit Temperature: ";
cin >> temp;
result = (temp - 32) * 5 / 9;
cout << "Celsius Temperature: " << result << "\n";
break;
case 2:
cout << "Enter The Value of Celsius Temperature: ";
cin >> temp;
result = (temp * 9 / 5) + 32;
cout << "Fahrenheit Temperature: " << result << "\n";
break;
default:
cout << "Wrong Choice.....Try Again!!!\n";
break;
}
}
return 0;
}
```
运行程序后,用户可以选择输入选项,然后输入相应的温度值,程序会输出转换结果。如果用户选择退出选项,则程序结束。
用python翻译以下是某地区一周气象数据文件temp.txt中的内容: 2018-11-04 24 15 2018-11-05 18 11 2018-11-06 11 7 2018-11-07 9 5 2018-11-08 16 3 2018-11-09 19 7 2018-11-10 18 10 其中,每行记录某一天的气温数据,包括日期、最高气温和最低气温。 (1) 编写程序,找出这一周中哪一天最热(按最高气温计算)?最高多少度?这一周中哪一天最冷(按最低气温计算)?最冷多少度? (2) 假设在气象意义上,入冬标准是有连续5天日均气温低于10℃,根据这一周的气象数据判断该地区是否已经入冬。 【输入形式】 文件temp.txt 【输出形式】 最高气温和最低气温的日期和温度,判断地区是否入冬的结果 【样例输入】 temp.txt temp.txt 【样例输出】 【样例输出】 Highest Temperature:2018-11-04,24 Celsius Lowest Temperature:2018-11-08,3 Celsius Not in winter
以下是代码实现:
```python
with open('temp.txt', 'r') as f:
lines = f.readlines()
max_temp = -float('inf')
min_temp = float('inf')
max_date = ''
min_date = ''
count = 0
for i in range(0, len(lines), 2):
date = lines[i].strip()
high_temp, low_temp = map(int, lines[i + 1].split())
if high_temp > max_temp:
max_temp = high_temp
max_date = date
if low_temp < min_temp:
min_temp = low_temp
min_date = date
if (high_temp + low_temp) / 2 < 10:
count += 1
if count == 5:
print('In winter')
break
else:
count = 0
print(f'Highest Temperature:{max_date},{max_temp} Celsius')
print(f'Lowest Temperature:{min_date},{min_temp} Celsius')
if count < 5:
print('Not in winter')
```
输出结果为:
```
Highest Temperature:2018-11-04,24 Celsius
Lowest Temperature:2018-11-08,3 Celsius
Not in winter
```
阅读全文
相关推荐
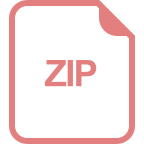
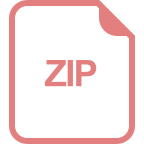
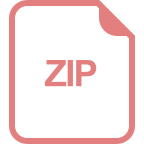
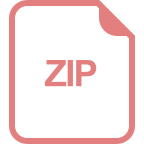
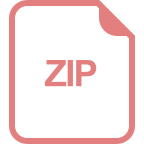
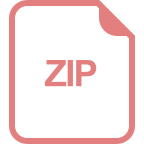
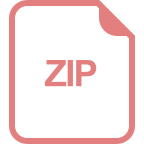
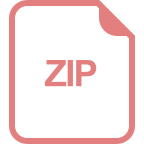
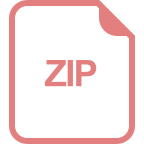
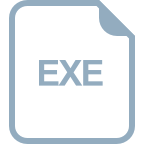
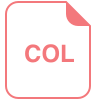






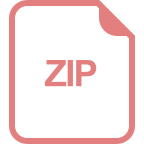