pytorch因果卷积
时间: 2023-11-07 14:06:00 浏览: 249
因果卷积是一种在时间序列数据或具有时序性的数据分析任务中应用的卷积操作。它引入了因果性,确保输出只依赖于过去的输入数据,不受未来信息的影响。因果卷积可以帮助我们建立模型来预测未来的数据,同时避免了信息泄露的问题。
膨胀因果卷积是在因果卷积的基础上引入了膨胀操作的一种卷积操作。它结合了因果卷积和扩张卷积的特性,能够增加感受野并保持因果性。通过膨胀操作,我们可以在保持计算效率的情况下获得更大的感受野。
在PyTorch中,可以使用torch.nn模块中的CausalConv1d类来实现因果卷积。该类提供了对时间序列数据进行因果卷积的功能。同时,PyTorch还提供了torch.nn.functional模块中的causal_conv1d函数,可用于在函数式编程环境中执行因果卷积操作。
相关问题
pytorch tcn
PyTorch TCN(Temporal Convolutional Network)是一个基于PyTorch框架的时间序列建模工具。它是一种使用卷积神经网络(CNN)进行时间序列建模的方法,具有很好的性能和灵活性。
TCN通过堆叠一系列的1D卷积层来对时间序列进行建模。这些卷积层具有不同的感受野(receptive field),可以捕捉到不同尺度的时间依赖关系。此外,TCN还使用了因果卷积(causal convolution)来确保模型对未来信息的依赖只通过之前的时间步骤进行。
PyTorch TCN可以用于很多时间序列应用,例如文本生成、语音识别、股票预测等。它提供了一个简单而强大的接口,可以轻松构建和训练TCN模型,并在实际应用中取得良好的效果。
你可以通过查阅PyTorch TCN的文档和示例代码来深入了解它的使用方法和技术细节。希望这个回答能解决你的问题!如果还有其他问题,请随时提问。
时空卷积网络python
时空卷积网络(Temporal Convolutional Network, TCN)是一种深度学习架构,特别适用于处理时序数据,如视频、音频和时间序列信号。在Python中,你可以使用深度学习库如Keras或PyTorch来实现TCN。下面是简要的介绍和实现步骤:
1. **原理**:
TCN通过堆叠多个1D卷积层并使用残差连接(Residual Connections)和可扩展的因果卷积(causal convolution)来捕捉长期依赖性。这些设计有助于避免梯度消失和过拟合。
2. **核心组件**:
- **1D卷积**:用于提取时序特征。
- **残差块(Residual Blocks)**:包含一个或多个1D卷积层,用于稳定训练过程。
- **扩张卷积(Dilated Convolution)**:增加感受野( receptive field)而不会增加计算复杂度。
- **归一化层(Normalization)**:如Batch Normalization,用于加速收敛。
3. **Python库实现**:
- **Keras**:可以使用`tf.keras.layers.Conv1D`和`TimeDistributed`等函数构建TCN层。
- **PyTorch**:`torch.nn.Conv1d`和`torch.nn.utils.rnn.PackedSequence`用于处理时序数据。
4. **示例代码片段**(简化版):
```python
from keras.models import Sequential
from keras.layers import Conv1D, LeakyReLU, Reshape, TimeDistributed
def create_tcn_block(filters, kernel_size, dilation_rate=1):
return [
Conv1D(filters, kernel_size, dilation_rate=dilation_rate),
LeakyReLU(),
Conv1D(filters, kernel_size, dilation_rate=dilation_rate)
]
model = Sequential()
model.add(TimeDistributed(Conv1D(64, 3), input_shape=(None, input_channels)))
model.add(Reshape((None, 64, 1))) # For residual connection
model.add(Conv1D(64, 3, padding='causal')) # First causal conv
for _ in range(num_blocks):
model.add(TimeDistributed(create_tcn_block(64, 3)))
```
**相关问题**:
1. 如何在实际项目中选择合适的TCN结构?
2. 如何在PyTorch中实现扩张卷积?
3. 使用TCN时,如何调整超参数以优化模型性能?
阅读全文
相关推荐
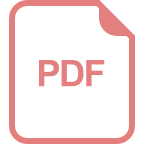
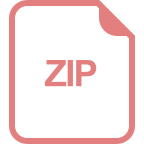
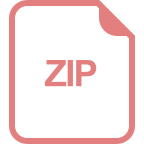
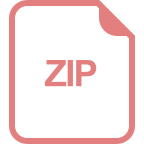
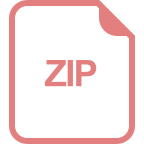
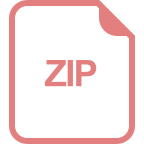
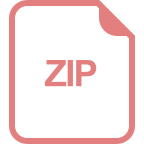
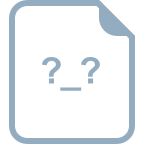
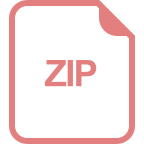
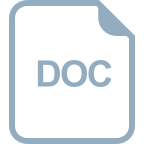
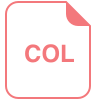