在 '__init__.py' 中找不到引用 'asarray'
时间: 2024-10-10 11:04:22 浏览: 32
在Python中,`__init__.py`是一个特殊的文件,它用于标记一个目录作为包(package)。当你遇到在`__init__.py`中找不到`asarray`的情况,这通常意味着以下几个问题:
1. **导入错误**:检查你的代码是否尝试从numpy或其他库中导入`asarray`函数,但路径或命名不正确。确保已经正确地安装了NumPy,并在import语句中使用正确的语法。
```python
from numpy import array # 或者 np.array
```
2. **模块结构问题**:`asarray`应该是`numpy`模块的一部分,如果这个函数在`__init__.py`内部使用而不是全局导入,那么你可能需要直接在相关的类或函数中导入。
3. **`numpy`不在当前作用域**:如果你的`__init__.py`文件不在包含numpy模块的同级目录下,你需要通过相对导入或改变工作目录来访问。
4. **误删除或覆盖**:确认`numpy`包及其功能没有被意外删除或替换,因为`asarray`是一个核心函数。
检查以上情况并相应地调整你的代码应该可以解决问题。如果问题依然存在,提供具体的代码段将有助于更好地定位问题。
相关问题
D:\Download\python.exe D:\study\作业\image\main.py 864 PY_VAR0 (500, 512, 3) Exception in Tkinter callback Traceback (most recent call last): File "D:\Download\lib\tkinter\__init__.py", line 1921, in __call__ return self.func(*args) File "D:\study\作业\image\main.py", line 311, in <lambda> button4 = Button(root2, text='执行程序', relief=GROOVE, font=('微软雅黑', 14),command=lambda:mediumbutton(img))#执行程序按钮 File "D:\study\作业\image\main.py", line 301, in mediumbutton img_medium1 = merge(img) File "D:\study\作业\image\main.py", line 297, in merge Label(image=zhifangtu2).place(x=670,y=50) File "D:\Download\lib\tkinter\__init__.py", line 3177, in __init__ Widget.__init__(self, master, 'label', cnf, kw) File "D:\Download\lib\tkinter\__init__.py", line 2601, in __init__ self.tk.call( _tkinter.TclError: image "[[[212 212 212] [212 212 212] [212 212 212] ... [212 212 212] [212 212 212] [212 212 212]] [[212 212 212] [212 212 212] [212 212 212] ... [212 212 212] [212 212 212] [212 212 212]] [[212 212 212] [212 212 212] [212 212 212] ... [212 212 212] [212 212 212] [212 212 212]] ... [[212 212 212] [212 212 212] [212 212 212] ... [212 212 212] [212 212 212] [212 212 212]] [[212 212 212] [212 212 212] [212 212 212] ... [212 212 212] [212 212 212] [212 212 212]] [[212 212 212] [212 212 212] [212 212 212] ... [212 212 212] [212 212 212] [212 212 212]]]" doesn't exist
这个错误信息的最后一行显示了一个很长的字符串,似乎是一个二维数组的字符串表示形式,它的格式看起来不正确。根据代码中的信息,这个字符串应该是一个图像的数组表示形式,但是它的格式不正确,所以在创建 `Label` 对象时出现了错误。
请确保在将图像数组传递给 `Label` 对象之前,将其转换为正确的图像对象。可以使用 `PIL` 库中的 `Image.fromarray` 方法将图像数组转换为 `PIL` 图像对象,然后再将其传递给 `Label` 对象的 `image` 属性。例如,可以这样转换图像数组并设置 `Label` 对象的图像:
```python
from PIL import Image, ImageTk
import numpy as np
import tkinter as tk
# 读取图像到数组中
img = cv2.imread('image.jpg')
# 将图像数组转换为 PIL 图像对象
img_pil = Image.fromarray(np.uint8(img))
# 将 PIL 图像对象转换为 Tkinter 图像对象
img_tk = ImageTk.PhotoImage(image=img_pil)
# 创建 Label 对象并设置图像
label = tk.Label(root, image=img_tk)
label.pack()
# 显示窗口
root.mainloop()
```
这样,应该就可以正确地显示图像了。
Exception in thread Thread-1: Traceback (most recent call last): File "D:\python\python3.7\lib\threading.py", line 926, in _bootstrap_inner self.run() File "D:\python\python3.7\lib\threading.py", line 870, in run self._target(*self._args, **self._kwargs) File "D:\python\python3.7\lib\tkinter\__init__.py", line 1489, in cget return self.tk.call(self._w, 'cget', '-' + key) TypeError: can only concatenate str (not "int") to str
这个错误是由于在使用 `tkinter` 中的 `Label` 组件时,传递给 `image` 参数的值应该是一个字符串,而不是一个整数。
在更新图像的代码中,你可能会遇到以下问题:
```python
photo = queue.get()
canvas.config(image=photo)
```
`config()` 方法的 `image` 参数需要传递一个字符串类型的值,而不是一个 `PhotoImage` 对象。为了解决这个问题,你可以使用实例变量来保存 `PhotoImage` 对象,然后在 `config()` 方法中使用该变量。
以下是修正后的代码示例:
```python
import tkinter as tk
import cv2
from PIL import Image, ImageTk
import threading
from queue import Queue
def start_camera(queue):
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
if not ret:
break
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
image = Image.fromarray(frame)
image = image.resize((640, 480))
photo = ImageTk.PhotoImage(image)
queue.put(photo)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
def update_frame(queue):
while True:
photo = queue.get()
canvas.image = photo # 保存 PhotoImage 对象到实例变量
canvas.config(image=canvas.image) # 使用实例变量作为参数
def start_thread():
queue = Queue()
camera_thread = threading.Thread(target=start_camera, args=(queue,))
frame_thread = threading.Thread(target=update_frame, args=(queue,))
camera_thread.start()
frame_thread.start()
root = tk.Tk()
canvas = tk.Label(root)
canvas.pack()
button = tk.Button(root, text="启动摄像头", command=start_thread)
button.pack()
root.mainloop()
```
在修正后的代码中,我们将 `PhotoImage` 对象保存到 `canvas.image` 实例变量中,在更新图像时使用该变量作为参数传递给 `config()` 方法,避免了类型错误。
阅读全文
相关推荐
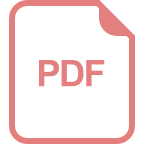
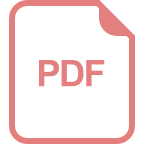
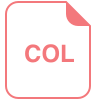
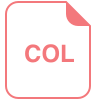
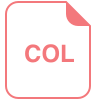
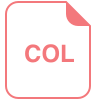
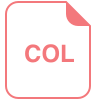
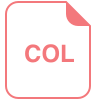
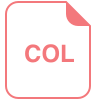
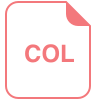
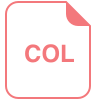




