编写程序,要求输入5个学生的成绩,输出学生间的最高分、平均分和最低分其中,输入成绩为带1位小数的浮点数,输出时要有文字说明,取小数点后2位数字。(允许使用math.h函数库)
时间: 2024-11-15 19:23:13 浏览: 65
编写程序来获取五名学生的成绩并计算最高分、平均分和最低分,可以按照以下步骤进行:
1. 首先,需要包含`stdio.h`和`math.h`头文件,以便使用输入输出和数学函数。
```c
#include <stdio.h>
#include <math.h>
```
2. 定义一个结构体来存储每个学生的姓名和成绩:
```c
typedef struct {
char name[20];
float score;
} Student;
```
3. 创建一个数组来存储学生的数据,并初始化它:
```c
Student students[5];
for (int i = 0; i < 5; i++) {
printf("请输入第 %d 学生的成绩:", i + 1);
scanf("%f", &students[i].score);
}
```
4. 接下来,我们需要找到最高分、最低分以及计算平均分:
```c
float max_score = students[0].score;
float min_score = students[0].score;
float total_score = 0;
// 找出最高分和最低分
for (int i = 1; i < 5; i++) {
if (students[i].score > max_score) {
max_score = students[i].score;
}
if (students[i].score < min_score) {
min_score = students[i].score;
}
// 累加总分数
total_score += students[i].score;
}
// 计算平均分
float average_score = total_score / 5.0f;
average_score = roundf(average_score * 100) / 100.0f; // 取两位小数并四舍五入
```
5. 最后,输出结果:
```c
printf("\n最高分:%.2f\n", max_score);
printf("平均分:%.2f\n", average_score);
printf("最低分:%.2f\n", min_score);
```
完整的代码示例:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
char name[20];
float score;
} Student;
int main() {
Student students[5];
for (int i = 0; i < 5; i++) {
printf("请输入第 %d 学生的成绩:", i + 1);
scanf("%f", &students[i].score);
}
float max_score = students[0].score;
float min_score = students[0].score;
float total_score = 0;
for (int i = 1; i < 5; i++) {
if (students[i].score > max_score) {
max_score = students[i].score;
}
if (students[i].score < min_score) {
min_score = students[i].score;
}
total_score += students[i].score;
}
float average_score = total_score / 5.0f;
average_score = roundf(average_score * 100) / 100.0f;
printf("\n最高分:%.2f\n", max_score);
printf("平均分:%.2f\n", average_score);
printf("最低分:%.2f\n", min_score);
return 0;
}
```
阅读全文
相关推荐
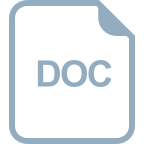
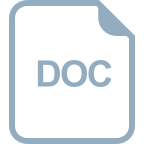
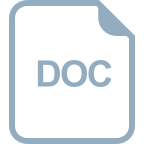














