这道题要求编写一个程序,利用友元函数实现比较学生的成绩。程序需要输入每个学生的学号、姓名、一门课的成绩等基本信息。可以计算出学生们的平均成绩,并输出所有学生中最高分和最低分的姓名。
时间: 2023-11-28 19:48:08 浏览: 47
好的,下面是一个简单的实现:
```cpp
#include<iostream>
#include<string>
using namespace std;
class Student {
private:
string id; // 学号
string name; // 姓名
double score; // 分数
public:
Student(string id, string name, double score) : id(id), name(name), score(score) {}
friend bool operator<(const Student& s1, const Student& s2); // 比较运算符的友元函数声明
friend bool operator>(const Student& s1, const Student& s2); // 比较运算符的友元函数声明
friend double average_score(Student* students, int n); // 计算平均分的友元函数声明
friend void find_max_min_score(Student* students, int n); // 查找最高分和最低分的姓名的友元函数声明
};
bool operator<(const Student& s1, const Student& s2) { // 比较运算符的友元函数定义
return s1.score < s2.score;
}
bool operator>(const Student& s1, const Student& s2) { // 比较运算符的友元函数定义
return s1.score > s2.score;
}
double average_score(Student* students, int n) { // 计算平均分的友元函数定义
double sum = 0;
for (int i = 0; i < n; i++) {
sum += students[i].score;
}
return sum / n;
}
void find_max_min_score(Student* students, int n) { // 查找最高分和最低分的姓名的友元函数定义
string max_name, min_name;
double max_score = students[0].score, min_score = students[0].score;
for (int i = 1; i < n; i++) {
if (students[i].score > max_score) {
max_score = students[i].score;
max_name = students[i].name;
}
if (students[i].score < min_score) {
min_score = students[i].score;
min_name = students[i].name;
}
}
cout << "最高分:" << max_name << " " << max_score << endl;
cout << "最低分:" << min_name << " " << min_score << endl;
}
int main() {
const int N = 3; // 学生数量
Student students[N] = {Student("001", "Tom", 80),
Student("002", "Jerry", 90),
Student("003", "Mike", 70)};
cout << "平均分:" << average_score(students, N) << endl;
find_max_min_score(students, N);
return 0;
}
```
在上面的实现中,我们定义了一个 `Student` 类,其中包含学号、姓名、分数三个成员变量。我们定义了两个友元函数 `operator<` 和 `operator>` 来比较两个学生的成绩大小,以便后续的排序和查找操作。我们还定义了两个友元函数 `average_score` 和 `find_max_min_score` 来计算平均分和查找最高分和最低分的姓名。在 `main` 函数中,我们创建了三个学生对象,并调用了这两个友元函数来进行计算和查找操作。
相关推荐
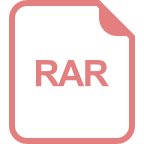
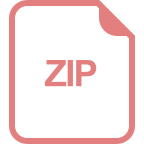







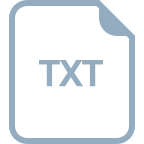
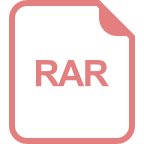
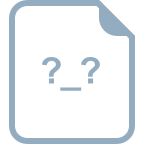
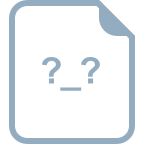
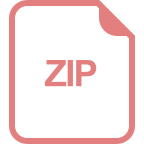
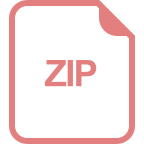