python的正则表达式匹配如何不区分大小写
时间: 2023-10-13 14:52:32 浏览: 138
要在Python中实现不区分大小写的正则表达式匹配,可以使用re模块的re.IGNORECASE标志。下面是一个示例:
```python
import re
pattern = r"hello"
text = "Hello, World!"
match = re.search(pattern, text, re.IGNORECASE)
if match:
print("匹配成功")
else:
print("匹配失败")
```
在上面的示例中,我们使用re.IGNORECASE标志来指定忽略大小写。这样,即使模式中的字母大小写不匹配,也能进行成功的匹配。在这个例子中,字符串"Hello, World!"中的"Hello"与模式"hello"匹配,因此会输出"匹配成功"。
相关问题
python正则表达式,不区分大小写
正则表达式是一种强大的文本处理工具,可以用来匹配、查找和替换文本中的特定模式。在Python中,我们可以使用re模块来使用正则表达式。如果你想要匹配不区分大小写的模式,可以使用re.IGNORECASE参数来实现。
举个例子,假设我们有一个字符串s = "Hello World",我们想要匹配其中的单词"hello",但不考虑大小写。我们可以使用如下代码来实现:
```
import re
s = "Hello World"
pattern = re.compile("hello", re.IGNORECASE)
result = pattern.search(s)
if result:
print("匹配成功")
else:
print("匹配失败")
```
在上面的代码中,我们使用re.compile()函数创建了一个正则表达式对象,然后指定了要匹配的模式为"hello",并且使用re.IGNORECASE参数表示忽略大小写。最后,我们使用search()函数在字符串s中查找匹配项,并输出结果。
Python正则表达式匹配忽略大小写
在 Python 中,正则表达式(Regular Expression)是一种强大的字符串处理工具,用于模式匹配和搜索。如果你想要进行不区分大小写的匹配,可以使用 `re.IGNORECASE` 或 `re.I` 标志。这里是如何操作的:
```python
import re
# 定义一个正则表达式模式,加上 re.IGNORECASE 或 re.I 标志
pattern = r"hello".lower() # 将要匹配的模式转换为小写,这样不区分大小写
case_insensitive_match = re.compile(pattern, re.IGNORECASE)
# 使用 compile 函数创建一个正则对象,并指定标志
# 或者直接在 match 或 search 函数中添加标志,如:
# match_insensitive = re.match(pattern + re.IGNORECASE, "Hello world")
# 进行匹配
text = "Hello World"
match = case_insensitive_match.search(text) # 或者 match_insensitive.search(text)
if match:
print("找到了匹配项:", match.group()) # 输出 "Hello"
else:
print("没有找到匹配项")
```
当你在搜索文本时,使用这个设置,Python 正则表达式会自动忽略匹配字符的大小写。
阅读全文
相关推荐
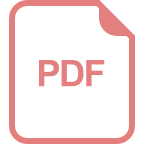
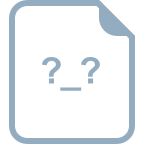
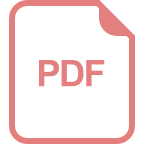
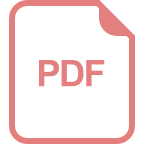
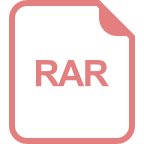
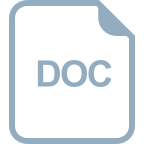
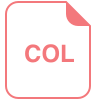


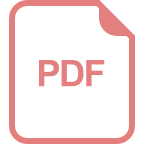
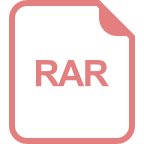
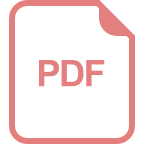
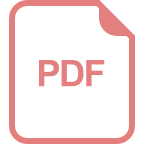
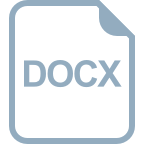
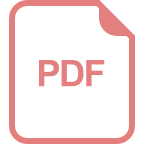
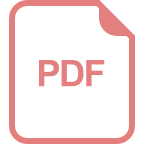
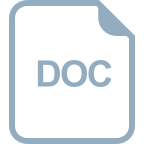