can you write a python script to download required packages for specific Mac OS and cpu version?
时间: 2024-11-05 16:33:42 浏览: 20
当然可以,Python有一个名为`pip`的包管理器,用于安装和管理软件包。如果你想编写一个脚本来根据特定的Mac OS版本和CPU类型下载所需的Python包,你可以这样做:
```python
import os
import subprocess
def detect_cpu_info():
command = "sysctl -n machdep.cpu.brand_string"
try:
cpu_brand = subprocess.check_output(command, shell=True).decode('utf-8').strip()
return cpu_brand
except Exception as e:
print(f"Failed to get CPU info: {e}")
return None
def get_macos_version():
command = "sw_vers -productVersion"
try:
mac_os_version = subprocess.check_output(command, shell=True).decode('utf-8').strip()
return mac_os_version
except Exception as e:
print(f"Failed to get macOS version: {e}")
return None
def install_package(package_name):
# For Python packages, use pip
command = f"pip3 install {package_name}"
# Check if we're on M1 chip first (since some packages might have separate wheels)
if is_m1_chip() and package_name.startswith("pytorch"):
# ... (add logic here for M1-specific package installation)
else:
subprocess.run(command, check=True)
def is_m1_chip():
return "Apple" in detect_cpu_info() and "M1" in detect_cpu_info()
macos_version = get_macos_version()
cpu_info = detect_cpu_info()
if macos_version and cpu_info:
package_to_install = input("Enter the name of the package to install: ")
install_package(package_to_install)
else:
print("Cannot determine system details.")
#
阅读全文
相关推荐
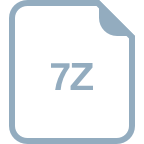
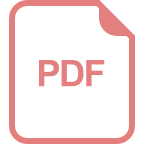
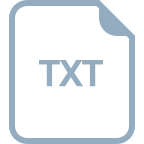
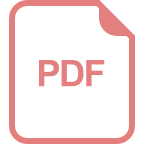
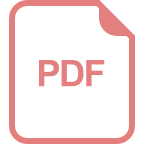
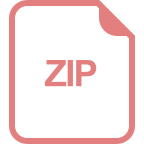
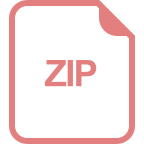
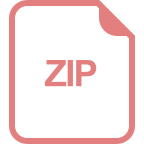
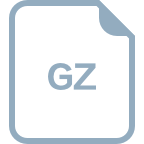
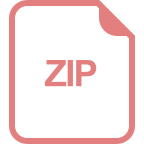
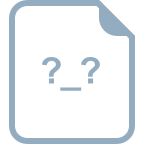
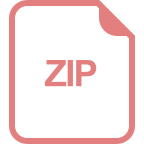
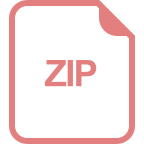
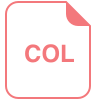
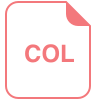
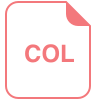

