线性表的顺序存储结构代码可建立,插入,删除,销毁,遍历,求长度
时间: 2024-10-17 08:07:23 浏览: 34
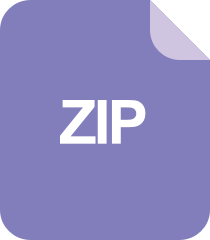
线性表的顺序存储与实现
线性表的顺序存储结构,也称为数组存储结构,是一种简单的数据结构,它将元素连续地存储在一段内存空间中。以下是基本操作的代码实现:
1. **创建(初始化)**:
```c++
int* createLinearList(int size) {
int* list = new int[size]; // 分配固定大小的内存
for (int i = 0; i < size; ++i) {
list[i] = 0; // 初始化所有元素
}
return list;
}
```
2. **插入(例如在开头)**:
```c++
void insertAtBegin(int* list, int value) {
int* newNode = new int; // 新节点
*newNode = value;
if (list) {
newNode[0] = list[0]; // 将第一个元素移动到新位置
delete[] list; // 释放原数组内存
}
list = newNode; // 更新指向新数组的指针
}
```
3. **删除(例如删除首元素)**:
```c++
void removeFirstElement(int* &list) { // 使用引用传递避免复制
if (list && list[0]) {
delete [] list; // 删除第一个元素并释放内存
list = list + 1; // 指向下一个元素,如果存在的话
} else {
cout << "List is empty or first element does not exist." << endl;
}
}
```
4. **遍历**:
```c++
void traverse(int* list) {
if (!list) {
cout << "List is empty." << endl;
} else {
for (int i = 0; list[i] != nullptr; ++i) {
cout << list[i] << " ";
}
cout << endl;
}
}
```
5. **求长度**:
```c++
int length(int* list) {
int count = 0;
while (list[count] != nullptr) {
++count;
}
return count;
}
```
6. **销毁(释放内存)**:
```c++
void destroyLinearList(int* list) {
delete[] list; // 释放整个数组的内存
}
```
阅读全文
相关推荐
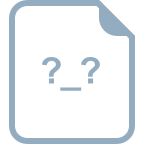
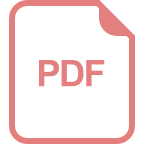
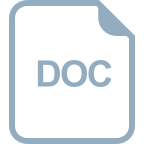
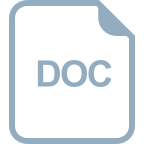
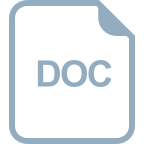
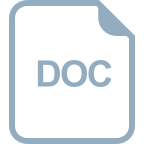
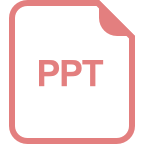
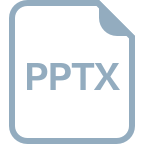
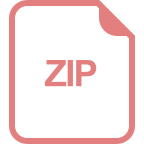
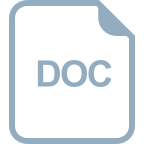
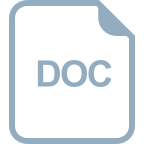
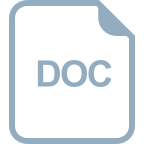
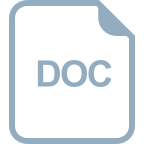
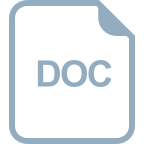
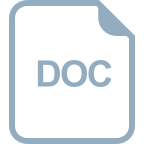
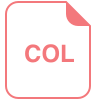

