用线性表实现一个通讯录
时间: 2024-10-15 15:19:01 浏览: 11
使用线性表(如数组、链表等数据结构)实现通讯录通常涉及到创建一个可以存储联系人信息的数据结构,每个联系人都包含姓名、电话号码、电子邮件等基本信息。下面是一个简单的示例,假设我们使用链表作为基础:
1. 定义一个联系人的数据结构,例如`Contact`类:
```python
class Contact:
def __init__(self, name, phone_number, email):
self.name = name
self.phone_number = phone_number
self.email = email
```
2. 创建一个`ContactList`类,使用链表存储联系人:
```python
class ContactList:
class Node:
def __init__(self, contact=None):
self.contact = contact
self.next = None
def __init__(self):
self.head = self.Node()
def add_contact(self, contact):
new_node = self.Node(contact)
if not self.head.contact:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
def find_contact(self, name):
current = self.head
while current and current.contact.name != name:
current = current.next
return current.contact if current else None
def search(self, keyword):
result = []
current = self.head
while current:
if keyword in current.contact.name or \
keyword in current.contact.phone_number or \
keyword in current.contact.email:
result.append(current.contact)
current = current.next
return result
```
阅读全文
相关推荐
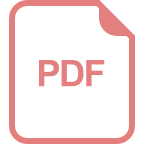
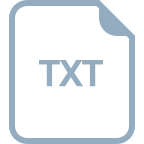
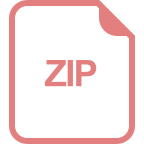















