编写函数,它的功能是:保留实数的n位小数,且最后一位进行四入五舍,返回该数。比如,1.235,保留两位小数后为 1.23。在main 数中验证。要求:实数和保留小数位数 n 在 main 函数中由键盘输入。注意是小于4进位大于5舍取
时间: 2024-09-09 21:07:10 浏览: 32
在 C 语言中,你可以使用 `double` 类型来处理实数,然后编写一个函数来根据指定的小数位数进行四舍五入。以下是一个简单的函数实现,名为 `round_to_n_places`:
```c
#include <stdio.h>
#include <math.h>
// 定义函数,接受一个double类型的参数和一个整数n,表示要保留的小数位数
double round_to_n_places(double num, int n) {
// 使用 floor() 函数确保结果不高于原数值(即四舍)
double result = floor(num * pow(10, n));
// 如果结果的小数部分大于等于 0.5,则加 1 后再除以 10 的 n 次方
if (fabs(result - trunc(result)) >= 0.5)
result += pow(10, -n);
return result / pow(10, n); // 返回四舍五入后的值
}
int main() {
double number;
int decimal_places;
printf("Enter a real number: ");
scanf("%lf", &number);
printf("Enter the number of decimal places to round to: ");
scanf("%d", &decimal_places);
// 验证并输出结果
double rounded_number = round_to_n_places(number, decimal_places);
printf("Rounded number with %d decimal places: %.2f\n", decimal_places, rounded_number);
return 0;
}
```
在这个程序中,`round_to_n_places` 函数首先乘以 10 的 n 次幂以便对每一位小数进行操作。如果四舍的结果加上小数部分大于或等于 0.5,就加 1。然后将结果除以 10 的 n 次幂,得到最终的四舍五入结果。
在 `main` 函数中,从用户那里获取输入的数字和要保留的小数位数,调用 `round_to_n_places` 并打印结果。请注意,`scanf` 和 `printf` 是用于输入和输出的库函数,`pow` 是计算幂次的数学函数。
阅读全文
相关推荐
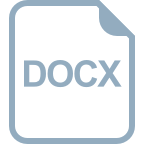
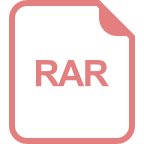
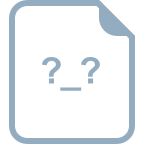















