司机注册,需要上传身份证,需要第三方识别身份证,然后还要把文件路径存入数据控如何实现代码java
时间: 2024-09-26 12:04:14 浏览: 40
在Java中实现司机注册功能,包括上传身份证照片、通过第三方API验证身份证并保存文件路径到数据库,可以按照以下步骤编写代码:
首先,你需要引入必要的库来处理图片上传和HTTP请求。例如,Spring Boot对文件上传支持良好,`HttpURLConnection` 或 `OkHttp` 可用于发送HTTP请求。
1. **设置环境**:
```java
import org.springframework.web.multipart.MultipartFile;
```
2. **创建上传接口** (假设在一个Controller中):
```java
@PostMapping("/register")
public ResponseEntity<?> registerDriver(@RequestParam("idCard") MultipartFile idCardFile) {
// 验证文件是否为空
if (idCardFile.isEmpty()) {
return new ResponseEntity<>(HttpStatus.BAD_REQUEST);
}
// 保存文件临时路径
String tempFilePath = saveTempFile(idCardFile);
// 使用第三方身份证识别服务
String isValid = checkIdCard(tempFilePath); // 假设checkIdCard是一个异步操作
// 如果身份证有效,将文件路径存入数据库
if (isValid) {
saveToDatabase(tempFilePath); // 假设saveToDatabase是一个持久化方法
// 返回成功响应
} else {
// 处理无效身份证
deleteTempFile(tempFilePath);
return new ResponseEntity<>("Invalid ID card", HttpStatus.FORBIDDEN);
}
}
```
3. **保存临时文件** 和 **删除临时文件**:
```java
private String saveTempFile(MultipartFile file) {
try {
File tempFile = new File("path/to/temp/directory/" + UUID.randomUUID() + ".jpg");
file.transferTo(tempFile);
return tempFile.getAbsolutePath();
} catch (IOException e) {
throw new RuntimeException("Failed to save temporary file", e);
}
}
private void deleteTempFile(String filePath) {
File tempFile = new File(filePath);
tempFile.deleteOnExit();
}
```
4. **调用第三方身份证验证API**:
```java
private String checkIdCard(String tempFilePath) {
// 假设这是一个异步方法,实际使用时替换为真实的网络请求
String response = makeHttpRequestToThirdPartyService(tempFilePath);
// 根据返回结果判断身份证是否有效
// ...
return isValid; // 假设isValid表示身份证有效性
}
// 这里只是一个示例,实际使用第三方库如OkHttp
private String makeHttpRequestToThirdPartyService(String tempFilePath) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://third-party-id-card-service.com/check")
.post(RequestBody.create(MediaType.parse("multipart/form-data"), tempFilePath))
.build();
try (Response response = client.newCall(request).execute()) {
if (!response.isSuccessful()) {
throw new IOException("Failed to verify ID card: " + response.body().string());
}
// 解析响应获取身份证验证结果
} catch (IOException e) {
throw new RuntimeException(e.getMessage(), e);
}
}
```
5. **数据库插入操作**:
```java
private void saveToDatabase(String filePath) {
// 实际连接数据库操作
jdbcTemplate.update("INSERT INTO drivers (id_card_path) VALUES (?)", filePath);
}
```
6. **错误处理和响应**:
记得添加适当的异常处理和状态码返回,以提供清晰的用户反馈。
阅读全文
相关推荐
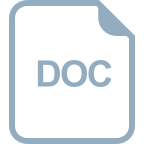
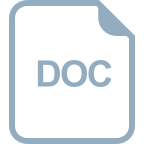
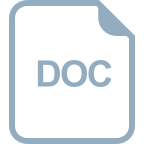
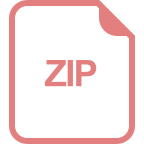
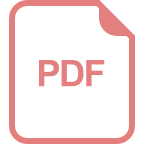
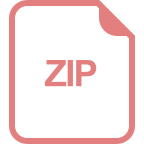
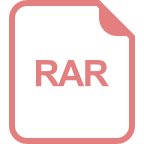
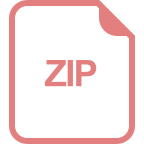
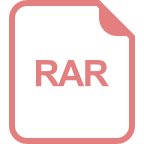
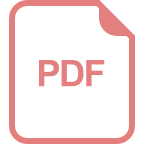
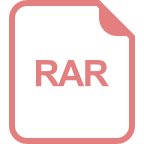
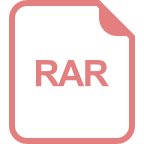
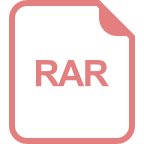
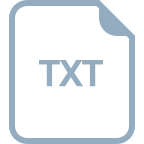
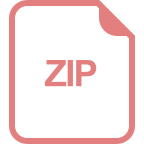