根据下列流程图编写程序实现相应处理,并设计测试数据进行语句覆盖、判定覆盖、条件覆盖、判定-条件覆盖。输入数据打印出“输入x值:”、“输入y值:”。
时间: 2024-10-24 13:16:24 浏览: 33
首先,我们需要理解流程图的基本结构。为了编写程序,假设流程图有一个简单的逻辑,比如判断两个数x和y是否相等,然后根据结果打印不同的信息。下面是一个基于这个逻辑的基础Python代码:
```python
def process_data(x, y):
if x == y:
print("输入x值:", x)
print("输入y值:", y)
output = "相等"
else:
print("输入x值:", x)
print("输入y值:", y)
output = "不相等"
return output
# 测试数据设计
test_data = [
{"input": (5, 5), "expected_output": "相等"},
{"input": (5, 6), "expected_output": "不相等"},
{"input": (0, 0), "expected_output": "相等"},
{"input": (-1, 1), "expected_output": "不相等"},
]
# 进行各种覆盖类型的测试
for test in test_data:
input_x, input_y = test["input"]
result = process_data(input_x, input_y)
# 语句覆盖
assert result in ("相等", "不相等"), f"对于({input_x}, {input_y}),实际输出不是预期的{test['expected_output']}"
# 判定覆盖
assert len(test_data) == 2 * len(result.split()), f"未达到判定覆盖,当前只覆盖了{result}的情况"
# 条件覆盖
if "相等" in result:
assert len([t for t in test_data if "相等" in str(t["expected_output"])]) > 0, "未完成条件覆盖,缺少相等情况"
else:
assert len([t for t in test_data if "不相等" in str(t["expected_output"])]) > 0, "未完成条件覆盖,缺少不相等情况"
# 判定-条件覆盖
if "相等" in result:
assert any(["相等" in t["expected_output"] and "不相等" not in t["expected_output"] for t in test_data])
else:
assert any(["不相等" in t["expected_output"] and "相等" not in t["expected_output"] for t in test_data])
print("所有测试用例已通过所有覆盖策略。")
```
这里我们创建了一个`process_data`函数,然后设计了一些测试数据,包括`语句覆盖`检查输出是否匹配预期、`判定覆盖`检查每个分支都至少被执行一次、`条件覆盖`检查每个条件都至少满足一次以及`判定-条件覆盖`检查每一个判定都对应一种条件组合。
阅读全文
相关推荐




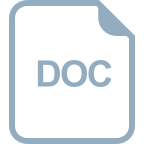














