filesystem c++11 删除目录下指定开头的文件
时间: 2024-12-09 20:16:23 浏览: 17
在C++11中,删除目录下的文件通常涉及到遍历目录并使用`std::filesystem`库来操作文件系统。如果你想删除所有以特定字符串开头的文件,你可以创建一个函数,如下所示:
```cpp
#include <iostream>
#include <filesystem>
void delete_files_with_prefix(const std::string& directory_path, const std::string& prefix) {
for (const auto & entry : std::filesystem::directory_iterator(directory_path)) {
if (entry.is_regular_file() && entry.path().filename().starts_with(prefix)) {
try {
std::cout << "Deleting file: " << entry.path() << std::endl;
std::filesystem::remove(entry.path());
} catch (const std::filesystem::filesystem_error &e) {
std::cerr << "Failed to delete file: " << e.what() << std::endl;
}
}
}
}
int main() {
std::string dir_to_search = "/path/to/your/directory";
std::string prefix_to_match = "file_"; // 想要删除的文件名前缀
delete_files_with_prefix(dir_to_search, prefix_to_match);
return 0;
}
```
在这个例子中,`delete_files_with_prefix`函数会查找给定目录`directory_path`下的所有以`prefix`开头的普通文件(不是目录),然后尝试使用`std::filesystem::remove`删除它们。
注意:
1. 一定要谨慎使用这个功能,因为删除操作是不可逆的,并且如果没有处理好异常,可能会导致数据丢失。
2. 使用`try-catch`块是为了捕获可能出现的错误,例如权限不足、路径不存在等。
3. 如果需要批量操作大量文件,可能要考虑使用更高级的文件操作策略,如并发处理。
阅读全文
相关推荐


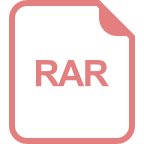

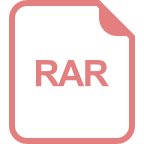








