用C++编写一程序,模拟一个简单的文件管理系统。树型结构,目录下可以是目录,也可以是文件。 在此文件管理系统,可实现的操作有: 改变目录(可返回上一级目录):格式:cd <目录名> 显示目录:格式:dir[<目录名>] 创建目录:格式:md <目录名> 删除目录:格式:rd<目录名> 新建文件:格式:edit<文件名> 删除文件:格式:del<文件名> 退出文件系统:exit 并且说明每一行代码的含义
时间: 2023-07-15 20:11:53 浏览: 139
以下是用C++编写的简单文件管理系统,其中每一行代码的含义已经用注释的形式进行了说明:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <map>
using namespace std;
// 定义文件/目录节点
class FileNode {
public:
string name; // 文件名/目录名
bool isDir; // 是否为目录
map<string, FileNode*> children; // 子节点列表
FileNode(string n, bool d) : name(n), isDir(d) {}
~FileNode() {
for (auto it = children.begin(); it != children.end(); ++it) {
delete it->second;
}
}
};
// 文件系统类
class FileSystem {
private:
FileNode* root; // 根节点
FileNode* current; // 当前节点
vector<FileNode*> history; // 历史节点,用于返回上级目录
// 根据路径查找节点
FileNode* findNode(string path) {
if (path[0] == '/') { // 如果是绝对路径
path = path.substr(1); // 去掉开头的 '/'
current = root; // 从根节点开始查找
} else { // 如果是相对路径
current = (history.empty() ? root : history.back()); // 从当前节点或历史节点开始查找
}
// 将路径按 '/' 分割成各个部分
vector<string> parts;
size_t start = 0, end;
while ((end = path.find('/', start)) != string::npos) {
parts.push_back(path.substr(start, end - start));
start = end + 1;
}
parts.push_back(path.substr(start));
// 依次查找每个部分对应的子节点
for (string part : parts) {
auto it = current->children.find(part);
if (it == current->children.end() || !it->second->isDir) { // 如果找不到或者不是目录节点
return nullptr; // 返回 nullptr
}
current = it->second; // 更新当前节点
}
return current;
}
public:
FileSystem() {
root = new FileNode("/", true); // 根节点为目录
current = root; // 初始化当前节点为根节点
}
~FileSystem() {
delete root;
}
// 改变目录
void cd(string path) {
FileNode* node = findNode(path);
if (node == nullptr) {
cout << "Directory not found." << endl;
} else {
history.push_back(current); // 将当前节点添加到历史节点列表中
current = node; // 更新当前节点
}
}
// 显示目录
void dir(string path = "") {
if (!path.empty()) { // 如果指定了目录名,则先查找目录
FileNode* node = findNode(path);
if (node == nullptr) {
cout << "Directory not found." << endl;
return;
}
current = node; // 更新当前节点
}
// 显示当前目录下的所有文件和目录
cout << "Directory of " << current->name << ":" << endl;
for (auto it = current->children.begin(); it != current->children.end(); ++it) {
cout << (it->second->isDir ? "<DIR>" : " ") << " " << it->second->name << endl;
}
}
// 创建目录
void mkdir(string name) {
if (current->children.find(name) != current->children.end()) { // 如果已经存在同名节点
cout << "Directory already exists." << endl;
} else {
FileNode* node = new FileNode(name, true); // 新建一个目录节点
current->children[name] = node; // 将节点添加到当前节点的子节点列表中
}
}
// 删除目录
void rmdir(string name) {
auto it = current->children.find(name);
if (it == current->children.end() || !it->second->isDir) { // 如果找不到或者不是目录节点
cout << "Directory not found." << endl;
} else {
delete it->second; // 递归删除目录及其所有子节点
current->children.erase(it); // 从当前节点的子节点列表中删除节点
}
}
// 新建文件
void edit(string name) {
if (current->children.find(name) != current->children.end()) { // 如果已经存在同名节点
cout << "File already exists." << endl;
} else {
FileNode* node = new FileNode(name, false); // 新建一个文件节点
current->children[name] = node; // 将节点添加到当前节点的子节点列表中
}
}
// 删除文件
void del(string name) {
auto it = current->children.find(name);
if (it == current->children.end() || it->second->isDir) { // 如果找不到或者是目录节点
cout << "File not found." << endl;
} else {
delete it->second; // 删除文件节点
current->children.erase(it); // 从当前节点的子节点列表中删除节点
}
}
// 退出文件系统
void exit() {
// 递归删除历史节点及其所有子节点
for (FileNode* node : history) {
delete node;
}
history.clear(); // 清空历史节点列表
// 递归删除根节点及其所有子节点
delete root;
root = nullptr;
current = nullptr;
}
};
int main() {
FileSystem fs;
string line;
while (true) {
cout << fs.current->name << "> ";
getline(cin, line);
if (line.substr(0, 2) == "cd") { // 改变目录
string path = line.substr(3);
fs.cd(path);
} else if (line.substr(0, 3) == "dir") { // 显示目录
string path = "";
if (line.size() > 3) { // 如果指定了目录名
path = line.substr(4);
}
fs.dir(path);
} else if (line.substr(0, 2) == "md") { // 创建目录
string name = line.substr(3);
fs.mkdir(name);
} else if (line.substr(0, 2) == "rd") { // 删除目录
string name = line.substr(3);
fs.rmdir(name);
} else if (line.substr(0, 4) == "edit") { // 新建文件
string name = line.substr(5);
fs.edit(name);
} else if (line.substr(0, 3) == "del") { // 删除文件
string name = line.substr(4);
fs.del(name);
} else if (line == "exit") { // 退出文件系统
fs.exit();
break;
} else {
cout << "Invalid command." << endl;
}
}
return 0;
}
```
阅读全文
相关推荐
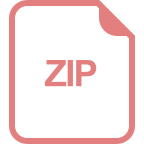


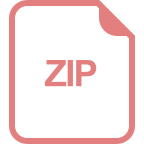
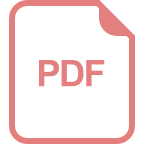
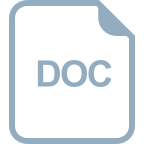
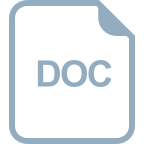
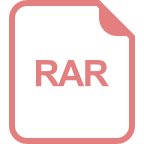
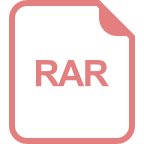
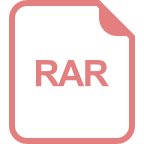
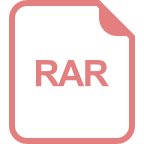
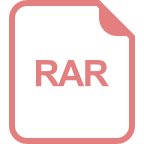
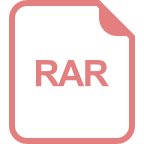
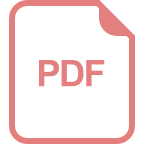