private Map<String, String> objToMap(Object o) { Map<String, String> map = new HashMap<>(); Class<?> aClass = o.getClass(); for (Field declaredField : aClass.getDeclaredFields()) { try { declaredField.setAccessible(true); Object o1 = declaredField.get(o); if (o1 == null || StringUtils.isBlank(o1.toString()) || StringUtils.equalsAny(declaredField.getName(), "accessToken", "memberId")) { continue; } map.put(declaredField.getName(), (String) o1); } catch (IllegalAccessException e) { log.error("出现异常", e); } finally { declaredField.setAccessible(false); } } return map; }
时间: 2024-02-14 08:31:17 浏览: 133
这段代码是一个将对象转换为Map的方法。它使用反射来获取对象的所有字段,并将字段名和字段值存储在一个Map中返回。
首先,它通过调用`o.getClass()`来获取传入对象的类。然后,使用`aClass.getDeclaredFields()`获取所有声明的字段。
接下来,通过循环迭代每个字段`declaredField`,设置`declaredField`为可访问(因为有些字段可能是私有的),然后通过调用`declaredField.get(o)`获取字段的值。
在获取字段值后,会进行一些条件判断:
- 如果字段值为`null`,或者为空白字符串,或者字段名等于"accessToken"或"memberId",则跳过该字段继续下一个字段的处理;
- 否则,将字段名和字段值存储在Map中,使用`map.put(declaredField.getName(), (String) o1)`。
最后,将存储了字段名和字段值的Map返回。
需要注意的是,在处理完每个字段后,通过`declaredField.setAccessible(false)`将字段设置为不可访问,以确保代码的安全性和正确性。
请问还有其他问题吗?
相关问题
public class TreeNode { private String name; private Map<String, TreeNode> children; public TreeNode(String name) { this.name = name; this.children = new HashMap<>(); } public String getName() { return name; } public Map<String, TreeNode> getChildren() { return children; } public void addChild(TreeNode child) { this.children.put(child.getName(), child); } public String toJson() { Gson gson = new GsonBuilder().setPrettyPrinting().create(); return gson.toJson(this); } public static TreeNode buildTree(Map<String, Map<String, Object>> data) { // 构建根节点 String rootName = (String) data.keySet().toArray()[0]; TreeNode root = new TreeNode(rootName); // 递归构建子树 buildSubtree(root, data.get(rootName)); return root; } private static void buildSubtree(TreeNode parent, Map<String, Object> data) { for (String childName : data.keySet()) { if (data.get(childName) instanceof Map) { // 如果子节点是一个 Map,则递归构建子树 Map<String, Object> childData = (Map<String, Object>) data.get(childName); TreeNode child = new TreeNode(childName); parent.addChild(child); buildSubtree(child, childData); } else { // 如果子节点不是一个 Map,则创建叶子节点 String leafName = (String) data.get(childName); TreeNode leaf = new TreeNode(leafName); parent.addChild(leaf); } } } }根据以上代码补全此方法:public static TreeNode buildTree(Map<String, Object> data) { }
根据代码中的实现,输入数据是一个Map对象,其中包含了树的节点信息,我们需要构建这个树并返回根节点。由于树的节点可以有多个子节点,我们可以使用Map来表示每个节点的子节点。
首先,我们需要定义一个节点类,包含节点的名称以及子节点的Map:
```
public class TreeNode {
private String name;
private Map<String, TreeNode> children;
public TreeNode(String name) {
this.name = name;
this.children = new HashMap<>();
}
public String getName() {
return name;
}
public Map<String, TreeNode> getChildren() {
return children;
}
public void addChild(TreeNode child) {
this.children.put(child.getName(), child);
}
}
```
然后,我们可以使用递归来构建这个树。具体地,我们可以从根节点开始构建,递归地构建子树。我们需要在输入数据中查找当前节点的子节点,如果存在则递归构建子树,否则将子节点设置为叶子节点。
```
public static TreeNode buildTree(Map<String, Object> data) {
// 构建根节点
String rootName = (String) data.keySet().toArray()[0];
TreeNode root = new TreeNode(rootName);
// 递归构建子树
buildSubtree(root, (Map<String, Map<String, Object>>) data.get(rootName));
return root;
}
private static void buildSubtree(TreeNode parent, Map<String, Map<String, Object>> data) {
for (String childName : data.keySet()) {
Map<String, Object> childData = data.get(childName);
if (childData instanceof Map) {
// 如果子节点是一个 Map,则递归构建子树
TreeNode child = new TreeNode(childName);
parent.addChild(child);
buildSubtree(child, (Map<String, Map<String, Object>>) childData);
} else {
// 如果子节点不是一个 Map,则创建叶子节点
String leafName = (String) childData;
TreeNode leaf = new TreeNode(leafName);
parent.addChild(leaf);
}
}
}
```
这个方法的时间复杂度是O(n),其中n表示树节点的个数。
封装一个resultMap,映射实体类public class EquipmentVo extends RegionalEquipment { private List<EquipmentDataVo> equipmentDataVoList; private List<Map<String,Object>> maps; public List<Map<String, Object>> getMaps() { return maps; } public void setMaps(List<Map<String, Object>> maps) { this.maps = maps; } public List<EquipmentDataVo> getEquipmentDataVoList() { return equipmentDataVoList; } public void setEquipmentDataVoList(List<EquipmentDataVo> equipmentDataVoList) { this.equipmentDataVoList = equipmentDataVoList; } },其中map中的键不固定,随着不同的SQL语句而改变,数据源是两个表,存在一对多关系
您可以封装一个resultMap来实现对EquipmentVo实体类的映射,具体实现可以参考以下代码:
```xml
<resultMap id="equipmentVoMap" type="EquipmentVo">
<result property="id" column="id"/>
<result property="name" column="name"/>
<result property="model" column="model"/>
<result property="regionId" column="region_id"/>
<!-- 一对多关系映射 -->
<collection property="equipmentDataVoList" ofType="EquipmentDataVo">
<result property="id" column="e_id"/>
<result property="name" column="e_name"/>
<result property="value" column="e_value"/>
<result property="equipmentId" column="equipment_id"/>
</collection>
<!-- 动态的map映射 -->
<collection property="maps" ofType="java.util.HashMap" resultMap="dynamicMap">
<id column="id" property="id"/>
<result column="name" property="name"/>
<result column="model" property="model"/>
<result column="region_id" property="regionId"/>
<result column="e_id" property="equipmentId"/>
<result column="e_name" property="equipmentName"/>
<result column="e_value" property="equipmentValue"/>
</collection>
</resultMap>
<resultMap id="dynamicMap" type="java.util.HashMap">
<result column="id" property="id"/>
<result column="name" property="name"/>
<result column="model" property="model"/>
<result column="region_id" property="regionId"/>
<result column="e_id" property="equipmentId"/>
<result column="e_name" property="equipmentName"/>
<result column="e_value" property="equipmentValue"/>
</resultMap>
```
其中,equipmentDataVoList是对应的一对多关系,maps是动态的map映射,ofType属性指定集合中元素的类型,resultMap属性指定使用的resultMap。动态的map映射需要额外定义一个resultMap,其中的property对应的是map中的键,column对应的是数据库表中的列名。在SQL语句中,需要使用AS关键字来指定map中的键名,例如:
```sql
SELECT e.*, d.id AS e_id, d.name AS e_name, d.value AS e_value
FROM equipment e
LEFT JOIN equipment_data d ON e.id = d.equipment_id
```
注意,当使用动态的map映射时,需要将实体类中对应的属性置为null,否则会出现重复映射的问题。
阅读全文
相关推荐
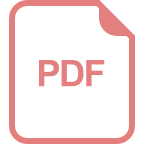
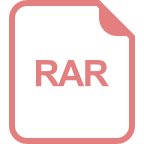
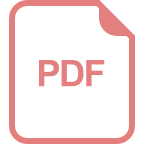













