List集合对象转为List<Map<String, Object>>
时间: 2024-01-01 16:21:06 浏览: 105
可以使用Java 8的Stream API将List集合对象转换为List<Map<String, Object>>。具体实现方法如下所示:
```java
import java.util.*;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
// 创建一个List集合对象
List<StudentInfo> studentList = new ArrayList<>();
studentList.add(new StudentInfo("Tom", 18, 175));
studentList.add(new StudentInfo("Jerry", 19, 180));
studentList.add(new StudentInfo("Mike", 20, 170));
// 将List集合对象转换为List<Map<String, Object>>
List<Map<String, Object>> mapList = studentList.stream()
.map(student -> {
Map<String, Object> map = new HashMap<>();
map.put("name", student.getName());
map.put("age", student.getAge());
map.put("height", student.getHeight());
return map;
})
.collect(Collectors.toList());
// 输出转换后的List<Map<String, Object>>
System.out.println(mapList);
}
}
class StudentInfo {
private String name;
private int age;
private int height;
public StudentInfo(String name, int age, int height) {
this.name = name;
this.age = age;
this.height = height;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public int getHeight() {
return height;
}
}
```
输出结果为:
```
[{name=Tom, age=18, height=175}, {name=Jerry, age=19, height=180}, {name=Mike, age=20, height=170}]
```
阅读全文
相关推荐
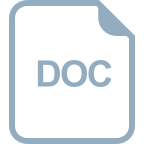
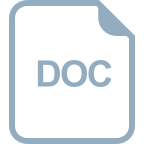
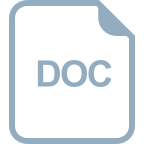















