判断tensor是否为空
时间: 2024-08-15 08:05:38 浏览: 157
判断Tensor是否为空主要依赖于使用的库以及特定情况下的定义,比如在PyTorch或TensorFlow等深度学习框架中。下面是一些基本的概念和步骤来进行这种判断:
### PyTorch示例:
在PyTorch中,你可以使用`.numel()`属性来获取张量中的元素总数,然后比较这个数是否等于0来确定张量是否为空。
#### 步骤说明:
1. **计算元素总数**:`tensor.numel()` 计算张量中总共有多少个元素。
2. **比较元素总数**:如果元素总数等于0,则表明张量是空的;如果不等于0,则张量含有数据。
#### 示例代码:
```python
import torch
# 创建一个空张量
empty_tensor = torch.tensor([])
# 非空张量
non_empty_tensor = torch.tensor([1, 2, 3])
# 检查是否为空
is_empty = (empty_tensor.numel() == 0)
is_non_empty = (non_empty_tensor.numel() != 0)
print("Empty tensor is", is_empty)
print("Non-empty tensor is", is_non_empty)
```
### TensorFlow示例:
在TensorFlow中,同样可以使用类似于PyTorch的方式来检查Tensor是否为空。
#### 步骤说明:
1. **检查形状是否全零**:由于`.shape`属性返回一个元组,可以逐维度检查是否全部为0。
2. **检查元素总数**:虽然不是直接使用`.size`,但是可以通过遍历形状的乘积来计算总的元素数,然后比较是否等于0。
#### 示例代码:
```python
import tensorflow as tf
# 创建一个空张量
empty_tensor = tf.constant([])
# 非空张量
non_empty_tensor = tf.constant([1, 2, 3])
# 检查是否为空
is_empty = tf.reduce_all(tf.equal(tf.shape(empty_tensor), ))
is_non_empty = tf.reduce_any(tf.not_equal(tf.shape(non_empty_tensor), ))
with tf.Session():
print("Empty tensor is", is_empty.eval())
print("Non-empty tensor is", is_non_empty.eval())
```
### 总结:
无论是在PyTorch还是TensorFlow中,判断Tensor是否为空的关键在于检查其中是否有元素存在。主要方法包括计算元素总数、检查形状是否全为零,或者利用各种布尔运算来简化判断过程。理解这些基础操作对处理大型模型训练和数据分析非常重要。
阅读全文
相关推荐
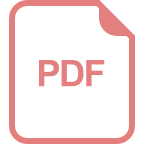
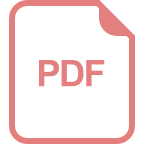
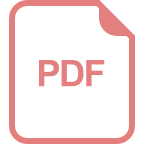















