删除字符串中的标点符号和多余的空格
时间: 2024-12-15 14:17:46 浏览: 3
删除字符串中的标点符号和多余空格通常是在数据清洗或文本预处理阶段的操作,目的是简化字符串以便于后续处理,比如分析或搜索。这个过程可以使用编程语言中的字符串操作函数或正则表达式来完成。以下是Python中的一种简单方法:
```python
import string
def remove_punctuation_and_spaces(s):
# 使用string.punctuation获取所有标点符号
punctuation = string.punctuation
# 使用replace()替换所有的标点符号为空
s = ''.join(c for c in s if c not in punctuation)
# 使用split()和strip()去除多余的连续空格
s = ' '.join(s.split())
return s
# 示例
input_string = "Hello, World! This is a test..."
cleaned_string = remove_punctuation_and_spaces(input_string)
print(cleaned_string) # 输出: Hello World This is a test
```
相关问题
删除字符串中标点符号和多余空格,用c语言编写程序
在C语言中,删除字符串中的标点符号和多余空格通常涉及到遍历字符串,并对字符逐个检查是否需要保留。这里我们可以使用ASCII码表来判断字符是否属于标点符号或空格。以下是一个简单的实现示例:
```c
#include <stdio.h>
#include <ctype.h> // 包含用于处理字符的库函数
// 功能:删除字符串中的标点符号和多余空格
void remove_punctuation_and_spaces(char *str) {
int i, j;
for (i = 0, j = 0; str[i] != '\0'; i++) { // 遍历字符串
if (!ispunct(str[i]) && !isspace(str[i])) { // 检查非标点和非空格字符
str[j++] = str[i]; // 保留并移动到新位置
}
}
str[j] = '\0'; // 结束字符串
}
int main() {
char str[100];
printf("请输入字符串:");
fgets(str, sizeof(str), stdin); // 从用户输入获取字符串
remove_punctuation_and_spaces(str);
printf("处理后的字符串:%s\n", str);
return 0;
}
```
删除字符串中的标点符号
删除字符串中的标点符号通常是为了处理文本数据,使其更适合于分析、搜索或清洗。你可以通过编程语言中的正则表达式或者内置函数来完成这个任务。以下是一个简单的Python示例:
```python
import string
def remove_punctuation(input_string):
# 定义包含所有标点符号的字符串
punctuation = string.punctuation
# 使用replace()函数将所有的标点替换为空格
cleaned_string = input_string.translate(str.maketrans('', '', punctuation))
return cleaned_string
# 示例
text_with_punctuation = "Hello, World!"
clean_text = remove_punctuation(text_with_punctuation)
print(clean_text) # 输出: Hello World
```
在这个例子中,`string.punctuation`包含了所有的标准ASCII标点符号,然后`str.maketrans()`创建了一个转换表,用于将输入字符串中的每个标点字符替换为空字符。
阅读全文
相关推荐
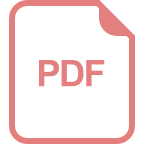
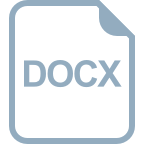
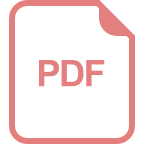












