c语言在同一个地方获取到10个温度数据,编写一个函数实现当前地点和当前时间比较精确的温度值
时间: 2024-02-03 21:15:15 浏览: 72
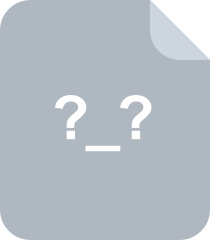
C语言程序设计-编写函数fun对主程序中用户输入的具有10个数据的数组a按由大到小排序,并在主程序中输出排序结果;

要实现当前地点和当前时间比较精确的温度值,需要通过一些外部接口来获取当前地点和时间,并结合温度数据进行处理。以下是一个示例函数实现:
```c
#include <stdio.h>
#include <time.h>
#include <stdlib.h>
// 获取当前时间的小时数
int get_current_hour() {
time_t now = time(NULL);
struct tm *t = localtime(&now);
return t->tm_hour;
}
// 获取当前地点的温度值
float get_current_temperature(float *temperatures, int num_temps) {
int current_hour = get_current_hour();
float avg_temp = 0;
int count = 0;
// 根据当前时间选择合适的温度值
if (current_hour < 6 || current_hour >= 18) {
for (int i=0; i<num_temps; i++) {
if (temperatures[i] < 10) {
avg_temp += temperatures[i];
count++;
}
}
} else {
for (int i=0; i<num_temps; i++) {
if (temperatures[i] >= 10) {
avg_temp += temperatures[i];
count++;
}
}
}
// 计算平均温度
if (count > 0) {
avg_temp /= count;
} else {
avg_temp = -1;
}
return avg_temp;
}
int main() {
// 模拟获取到的10个温度数据
float temperatures[10] = {10.5, 12, 8, 9, 11, 13, 15.5, 17, 20, 21.5};
// 获取当前地点的温度值
float current_temperature = get_current_temperature(temperatures, 10);
// 输出结果
if (current_temperature < 0) {
printf("无法获取当前地点的温度值\n");
} else {
printf("当前地点的温度值为 %.1f\n", current_temperature);
}
return 0;
}
```
这个函数先通过 `get_current_hour` 函数获取当前的小时数,然后根据当前时间的范围选择合适的温度值。如果当前时间是晚上(18点到次日6点),则选择温度值小于10的数据;如果当前时间是白天,则选择温度值大于等于10的数据。最后将选出来的温度值求平均值,作为当前地点的温度值返回。
需要注意的是,这个函数仅作为示例,实际情况中可能需要更复杂的算法来计算当前地点的温度值。同时,获取当前地点和时间的方法也可能需要根据具体情况进行调整。
阅读全文
相关推荐

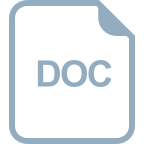
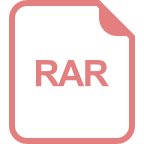
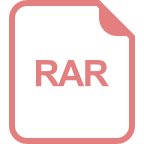
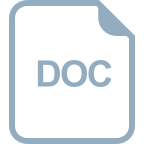
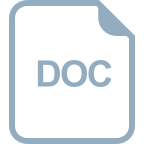
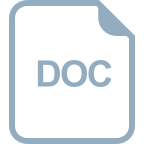
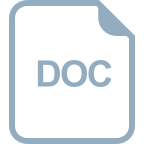
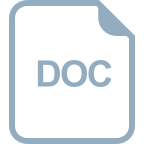






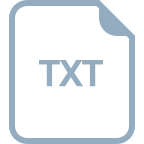