在gateway中调用feign
时间: 2023-10-14 19:04:57 浏览: 144
在使用 Feign 进行服务调用时,通常需要在 Spring Boot 应用中配置 FeignClient 接口的实现类。而在 Spring Cloud Gateway 中,我们可以通过配置一个 Filter 来实现 FeignClient 的调用。
具体步骤如下:
1. 首先,在 pom.xml 文件中加入 Feign 的依赖:
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
```
2. 在应用程序的启动类上添加 `@EnableFeignClients` 注解,以启用 Feign 客户端功能。
```java
@SpringBootApplication
@EnableFeignClients
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
```
3. 创建一个 FeignClient 接口,用于定义需要调用的服务。例如:
```java
@FeignClient(name = "test-service")
public interface TestClient {
@GetMapping("/hello")
String hello();
}
```
4. 创建一个 Filter,用于将请求转发给 FeignClient 接口。例如:
```java
@Component
public class FeignFilter implements GlobalFilter, Ordered {
private final TestClient testClient;
public FeignFilter(TestClient testClient) {
this.testClient = testClient;
}
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
String result = testClient.hello();
ServerHttpResponse response = exchange.getResponse();
response.setStatusCode(HttpStatus.OK);
response.getHeaders().add(HttpHeaders.CONTENT_TYPE, MediaType.APPLICATION_JSON_VALUE);
byte[] bytes = result.getBytes(StandardCharsets.UTF_8);
DataBuffer buffer = response.bufferFactory().wrap(bytes);
return response.writeWith(Flux.just(buffer));
}
@Override
public int getOrder() {
return -1;
}
}
```
在这个 Filter 中,我们注入了上面定义的 TestClient 接口,并在 filter 方法中调用了该接口的 hello 方法。最后,我们将返回的结果写回给客户端。
5. 在 application.yml 文件中添加 FeignClient 的配置:
```yaml
spring:
application:
name: gateway
feign:
client:
config:
default:
loggerLevel: full
```
在这个配置中,我们将 FeignClient 的日志级别设置为 `full`,以方便调试。
6. 启动应用程序,并访问 Gateway 的 URL,即可看到 FeignClient 的调用结果。
阅读全文
相关推荐
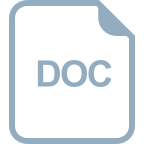
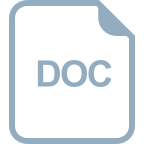
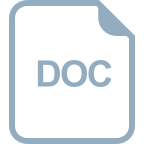

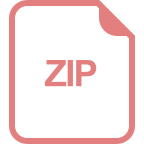



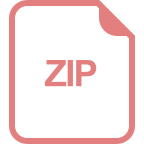
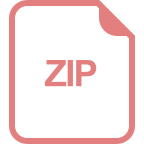
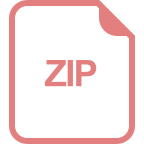
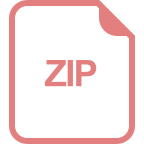
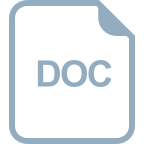





