答辩问使用spring boot和 vue如何实现商品的销量排行
时间: 2023-06-26 08:09:55 浏览: 252
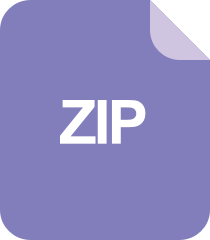
spring boot + Vue 实现汽车销售管理系统

使用Spring Boot和Vue实现商品的销量排行可以通过以下步骤实现:
1. 在Spring Boot后端中,建立一个API接口,用于获取商品销量排行榜数据。可以使用Spring Data JPA框架与数据库进行交互,查询出销量前几的商品数据,并返回给前端。
2. 在Vue前端中,使用axios等工具调用后端提供的API接口,获取销量排行榜数据,并将其展示在页面上。
3. 在Vue前端中,使用echarts等图表库,将销量排行榜数据以柱状图等形式进行可视化展示。
4. 可以使用定时任务等方式,定期更新后端数据库中的销量数据,以保证销量排行榜数据的准确性。
总的来说,使用Spring Boot和Vue实现商品的销量排行需要后端和前端两个部分的配合,其中后端主要负责提供API接口和与数据库交互,前端则主要负责展示数据和图表。
阅读全文
相关推荐
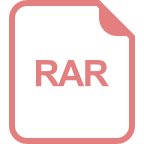






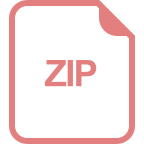
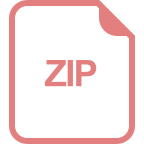