答辩问使用spring boot和 vue如何商品是否推荐
时间: 2023-06-26 14:09:51 浏览: 113
使用 Spring Boot 和 Vue 可以开发一个完整的电商网站,包括商品推荐功能。具体的实现方式可以是:
1. 在后端使用 Spring Boot 搭建一个 RESTful API 服务,提供商品管理和推荐功能的接口。
2. 在前端使用 Vue 实现一个商品推荐的页面,通过调用后端提供的接口获取推荐商品的数据。
3. 在后端实现商品推荐功能时,可以使用机器学习算法来根据用户的历史浏览记录、购买记录、收藏等信息进行推荐。也可以使用基于规则的推荐算法,根据商品的属性、标签等信息进行推荐。
4. 在前端实现商品推荐页面时,可以使用 Vue 的组件化开发方式,将推荐商品展示组件化,方便复用和维护。
5. 在前端实现商品推荐页面时,可以使用 Vue 的路由功能,实现多个推荐页面之间的跳转和切换。
6. 在前端实现商品推荐页面时,可以使用 Vue 的数据绑定和事件绑定功能,实现用户对推荐商品的交互操作,如添加到购物车、查看详情等。
综上所述,使用 Spring Boot 和 Vue 可以很好地实现商品推荐功能,提高电商网站的用户体验和营收效果。
相关问题
答辩问使用spring boot和 vue如何商品是否推荐在数据库中查询商品推荐的状态如何查询条件是什么代码
在使用Spring Boot和Vue开发电商平台时,商品推荐的状态通常会存储在数据库中,如MySQL或MongoDB。要查询推荐状态,可以使用Spring Data JPA或Spring Data MongoDB等框架进行数据库操作。在Vue中,可以通过调用后端API来获取商品的推荐状态。
查询条件通常包括商品ID、用户ID和推荐类型等。以下是一个示例代码片段,用于从MySQL数据库中查询商品推荐状态:
```java
@Repository
public interface ProductRecommendationRepository extends JpaRepository<ProductRecommendation, Long> {
List<ProductRecommendation> findByProductIdAndUserIdAndRecommendationType(Long productId, Long userId, RecommendationType recommendationType);
}
```
在上面的代码中,`ProductRecommendation`是一个实体类,表示商品推荐的状态。`findByProductIdAndUserIdAndRecommendationType`方法使用Spring Data JPA提供的命名约定来查询符合条件的商品推荐状态。其中,`productId`表示商品ID,`userId`表示用户ID,`recommendationType`表示推荐类型。
在Vue中,可以通过Axios库调用后端API来获取商品推荐状态。以下是一个示例代码片段:
```javascript
import axios from 'axios';
export default {
data() {
return {
recommendation: null
}
},
methods: {
getProductRecommendation(productId, userId, recommendationType) {
axios.get(`/api/product/recommendation?productId=${productId}&userId=${userId}&recommendationType=${recommendationType}`)
.then(response => {
this.recommendation = response.data;
})
.catch(error => {
console.log(error);
});
}
}
}
```
在上面的代码中,`getProductRecommendation`方法使用Axios库调用后端API来获取商品推荐状态。其中,`productId`、`userId`和`recommendationType`是查询条件。在获取到推荐状态后,将其保存在Vue组件的`recommendation`属性中,以便在页面中显示。
答辩问使用spring boot和 vue如何实现商品的销量排行如何对销量进行排序
使用Spring Boot和Vue实现商品的销量排行,可以通过以下步骤来实现:
1. 在Spring Boot后端中定义商品销量的属性,并在数据库中存储每个商品的销量信息。
2. 在后端中定义API接口,用于获取所有商品的销量信息,并按照销量进行排序。
3. 在Vue前端中,通过调用后端API接口获取所有商品的销量信息,并将其显示在页面上。
4. 在Vue前端中,使用Vue的排序功能对商品销量进行排序,并将排好序的商品列表显示在页面上。
代码示例:
后端代码(Java + Spring Boot):
```java
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private int sales; // 商品销量
// 省略getter和setter方法
}
@RestController
@RequestMapping("/api/products")
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping
public List<Product> getAllProducts() {
return productService.getAllProducts();
}
}
@Service
public class ProductService {
@Autowired
private ProductRepository productRepository;
public List<Product> getAllProducts() {
return productRepository.findAll();
}
}
public interface ProductRepository extends JpaRepository<Product, Long> {
}
```
前端代码(Vue):
```html
<template>
<div>
<h2>商品销量排行榜</h2>
<table>
<thead>
<tr>
<th>商品名称</th>
<th>销量</th>
</tr>
</thead>
<tbody>
<tr v-for="product in sortedProducts">
<td>{{ product.name }}</td>
<td>{{ product.sales }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
products: []
}
},
created() {
axios.get('/api/products')
.then(response => {
this.products = response.data
})
},
computed: {
sortedProducts() {
return this.products.slice().sort((a, b) => b.sales - a.sales)
}
}
}
</script>
```
阅读全文
相关推荐
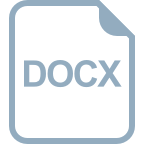
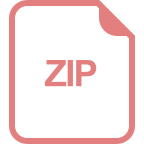









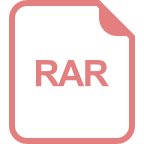
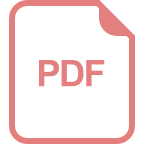
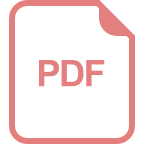
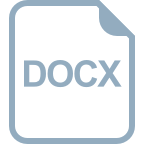
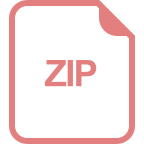